Rust Programming Language: Memory Safety and Performance Explained
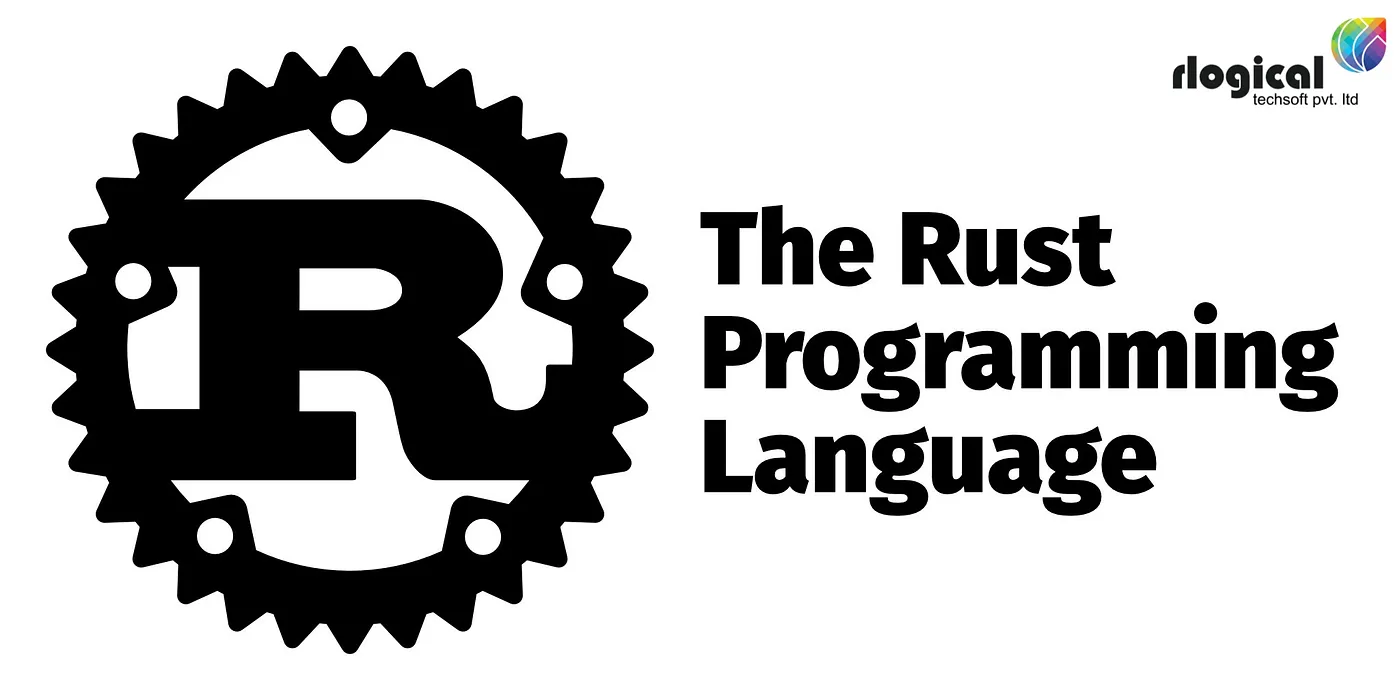
Written by Massa Medi
Rust programming language is revolutionizing the way developers build high performance, memory safe applications. Renowned for its blend of modern simplicity and low-level power, Rust has quickly become the go to choice for everything from game engines to operating systems and even WebAssembly targets. In this comprehensive guide, we’ll explore exactly what sets Rust apart, how its unique memory management model works, and why its passionate community of “Rustaceans” keeps voting it the most beloved programming language year after year.
What is the Rust Programming Language?
The Rust language stands at the intersection of performance and safety. Unlike typical high-level languages that use garbage collectors to handle memory, Rust manages to keep its promises of memory safety while delivering close to the metal performance. This makes it an attractive choice for critical systems such as databases, operating systems, and performance intensive applications where every nanosecond counts.
Rust’s journey began in 2007 as a personal project by Graydon Hoare, who named it after the resilient rust fungus. With the support of Mozilla starting in 2009, Rust rose from niche experiment to a central tool for cutting edge software engineering. Since 2016, the Rust community affectionately known as Rustaceans has consistently voted it the most loved programming language according to the yearly Stack Overflow Developer Survey.
Memory Management in Rust: No Garbage Collector, No Compromises
Traditional high-level programming languages, like Python or Java, often rely on garbage collectors to automatically reclaim unused memory. While this approach is convenient, it comes with performance tradeoffs and less direct control for the developer. Lower-level languages, such as C or C++, hand over memory management through manual calls to functions like malloc
, free
, new
, or delete
, increasing both flexibility and risk for catastrophic bugs think memory leaks, dangling pointers, or undefined behavior.
Rust charts a unique path. It has no garbage collector. Instead, it achieves memory safety through a concept called ownership and borrowing. This innovation is at the very heart of Rust's appeal.
Understanding Rust’s Ownership and Borrowing System
Immutable by Default
In Rust, every variable is immutable by default. This means that unless explicitly specified otherwise, the values you define cannot be changed after their initial assignment. This approach encourages a safer coding style and enables many performance optimizations.
Stack vs Heap: Where Values Live
- Stack Memory: Used for simple, fixed size data types. Access is extremely fast, with very little overhead.
- Heap Memory: Required for mutable data or dynamically sized objects. While more flexible, it incurs additional performance costs.
What is Ownership?
Every value in a Rust program is assigned a single “owner” usually a variable. When this variable goes out of scope (for instance, when a function finishes executing), Rust automatically calls the drop
method and releases any associated memory. This eliminates the need for manual memory management and prevents a broad class of bugs.
Borrowing: Sharing Without Surrendering
Sometimes, a different part of the program needs to access a value without taking ownership. Rust’s borrowing system allows this by letting variables temporarily “lend out” a reference to their data. These rules are enforced at compile time using the famed borrow checker which ensures references cannot outlive their owners or introduce data races.
For example, let’s say you have a variable storing a string. You want another function to read this string but not modify or take control over it. With borrowing, you can safely pass a reference (using an ampersand &
), and Rust guarantees both safety and performance. All borrowing rules are checked before your code is ever run, preventing common pitfalls like dangling pointers or unsafe memory access.
How Does the Borrow Checker Work?
The borrow checker validates your code according to strict but well designed rules:
- You can have either one mutable reference or any number of immutable references to a value at a time (not both simultaneously).
- References must always be valid; they cannot outlive the data they point to.
If you violate these rules, the compiler stops you with a helpful error before the program ever runs. This system strikes a careful balance between performance and memory safety making Rust popular for mission critical systems that cannot afford bugs.
Building and Managing Rust Projects with Cargo
Rust's ecosystem is powered by its robust package manager, Cargo. In Rust vocabulary, each individual package is known as a crate. Cargo not only makes it easy to manage dependencies, build projects, and run tests, but it also empowers the community to share and discover reusable code.
Setting Up Your First Rust Project
- Install Rust using the recommended installer from rust-lang.org.
- Initialize a New Project: Run
cargo new my_project
in your terminal. - Main Execution Entry Point: Open the
src/main.rs
file you’ll see a defaultfn main()
function, where program execution begins.
Declaring Variables in Rust
To declare a variable, use the let
keyword, followed by the variable's name and type:
let my_variable: i32 = 10;
By default, variables are immutable. To make them mutable, add mut
:
let mut counter: i32 = 0;
The variable acts as the "owner" of the value. If you need to borrow its location in memory, prefix with an ampersand:
let number_ref = &counter;
To print data to standard output, use the println! macro:
println!("The counter is {}", counter);
Rust’s Standard Library and Building Executables
Rust includes a powerful standard library that covers a wide array of features:
- Input/Output (IO) management
- File system manipulation
- Concurrency tools
- Collections and algorithms
To compile your code into a fast native executable, simply invoke the Rust compiler automatically handled by Cargo with cargo build
or cargo run
.
With just a few simple steps, you’ve created a small, robust, and memory safe executable perfect for resource intensive requirements and applications where reliability and speed matter most.
Why Rust Is Loved by Developers Worldwide
Rust’s unique combination of memory safety, performance, and robust tooling has earned it a fiercely loyal fanbase. The fact that it is routinely crowned the “most loved language” in developer polls is no accident. By empowering developers with tools that catch mistakes at compile time and freeing them from entire categories of runtime bugs Rust allows you to build futuristic applications today.
Whether you’re working on a next gen game engine, a lightning-fast database, or future proofing your WebAssembly strategy, mastering Rust will give you an edge in modern software engineering.
Frequently Asked Questions about Rust Programming Language
What is Rust best used for?
Rust is ideal for building high-performance, reliable software such as operating systems, embedded systems, game engines, databases, web servers, and projects targeting WebAssembly. Its memory safety and concurrency guarantees make it a top choice for mission critical applications.
How does Rust ensure memory safety without a garbage collector?
Rust’s ownership and borrowing system, enforced at compile time by the borrow checker, ensures that every piece of data has a single owner and that references to data cannot outlive their owners. This approach prevents memory leaks and data races, delivering memory safety without the runtime overhead of garbage collection.
What is Cargo and what are crates in Rust?
Cargo is Rust’s official package manager and build system. It simplifies project creation, dependency management, compilation, and testing. A crate is the Rust term for a package or library module a single deployable unit that can be shared or reused across projects.
Why are variables immutable by default in Rust?
Rust encourages immutability to help prevent unintentional bugs and make code easier to reason about. By defaulting variables to immutable, Rust helps you write safer code, reducing side effects and making concurrent programming less error prone.
How do you make a variable mutable in Rust?
To declare a mutable variable, add the mut
keyword when using the let
statement. For example: let mut value = 5;
Now, you can reassign a different value to value
as needed.
What is the Rust borrow checker?
The borrow checker is a component of the Rust compiler that enforces the rules of ownership and borrowing. It checks for invalid or unsafe references and stops compilation if it detects potential memory errors, such as dangling pointers, race conditions, or use after free issues.
Is Rust good for beginners?
Rust’s learning curve is steeper than that of some scripting languages, especially due to its strict memory safety features. However, its helpful compiler errors, excellent documentation, and supportive community make it an excellent choice for motivated beginners interested in systems programming.
Is Rust better than C++?
Both Rust and C++ have strengths and fit different contexts. Rust’s memory model and safety guarantees reduce bugs and undefined behavior common in C++ codebases, making it better suited for new projects prioritizing safety, concurrency, and reliability. However, C++ boasts a vast legacy ecosystem and may still be preferred for certain legacy or platform-specific requirements.