Computer Science Basics: A Beginner’s Guide to 101 Essential Terms and Concepts
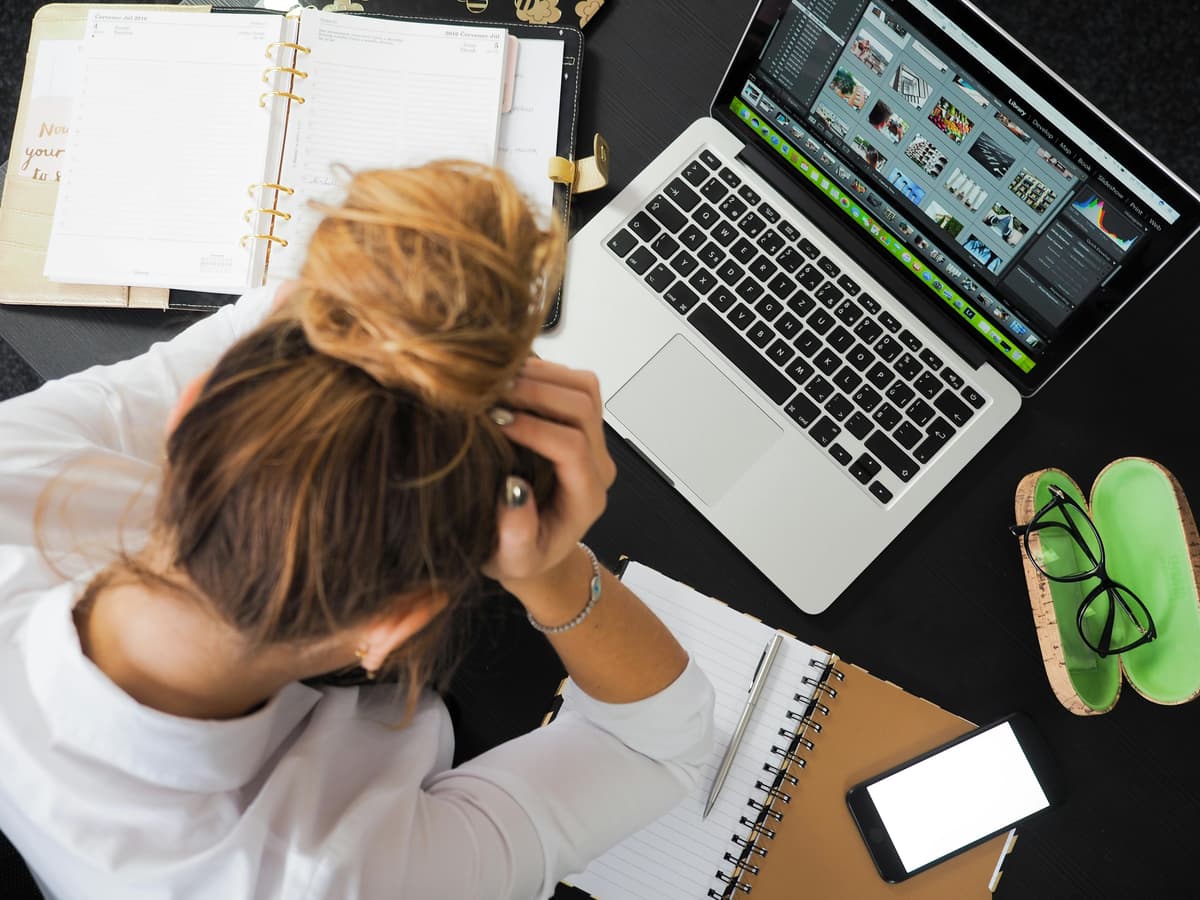
Written by Massa Medi
Wondering what to do when your code throws a cryptic error? The universally accepted strategy is, of course, to change absolutely nothing and mash the “run” button a few more times in pure, hopeful desperation. If that fails no fear! You’ll just need to acquire a computer science degree, right? (Spoiler: you absolutely do not.)
Welcome to the whimsical magic that is software engineering: a field where you can wield the power to create groundbreaking applications, land lucrative jobs, and be trusted to pilot a technological “metal tube” at 30,000 feet… all while feeling that everything is held together by invisible tech glue. Today, pull up a (virtual) chair for Computer Science 101, where we’re breaking down 101 computer science terms and concepts that will transform your “garbage code” into a masterpiece or at least help you explain to Grandma why her printer isn’t working.
What Really *Is* a Computer?
A computer, stripped down to its most basic form, is more primitive than you might think. Imagine a long strip of tape that stores sequences of ones and zeros. This, coupled with a simple device that can read (and write) those numbers, forms what’s called a Turing Machine. In tinkerer’s theory, this humble machine can compute anything from the awe inspiring graphics on your favorite game to the mysterious algorithms that led you to this very article.
At the heart of real-world computers lies the Central Processing Unit (CPU). Bust one open (not recommended for actual hardware), and you'll find a baffling array of billions of minuscule transistors tiny on-off switching gates for electrical signals. Each switch can be either a 0 or a 1, known as a bit the foundational unit of information in computing.
But a solo bit is hardly more useful than a single grain of rice. What you’re really working with are groups of 8 bits, known as a byte. Each byte can represent 256 different values enough to encode every letter, number, and symbol on your keyboard. In fact, when you type a character, it’s mapped to a binary number through encoding systems like ASCII or UTF-8.
Binary is computers’ native tongue: a base-2 system of only 1s and 0s, akin to the decimal (base-10) system you use when counting on fingers just a little less ergonomic. Since reading a long string of 1s and 0s would make anyone’s eyes cross, engineers represent them more palatably with hexadecimal (base-16). It takes 0–9, then throws in A–F, to conveniently express “nibbles” (4-bit chunks).
Programming, Machine Code, and Memory Explained
When you write code say, in Python or JavaScript your clever logic is eventually morphed into machine code: binary instructions that your CPU knows how to run directly.
Random Access Memory (RAM)
But where does your data *live* as your program runs? Enter Random Access Memory, or RAM. Imagine RAM as a neighborhood where each house contains a byte, and every byte’s “address” lets your computer fetch and store events at lightning speed.
Together, the CPU and RAM are your computer’s brain the fast thinking, high-speed part of hardware.
Handling Input and Output (I/O)
Of course, a computer without input and output is just a very expensive brick. Input devices (keyboards, mice) let you communicate with your device, while output devices (monitors, speakers) broadcast the results.
Thankfully, you don’t need to wire these pieces together every time. Operating system kernels (think Windows, MacOS, Linux) handle hardware management using something called device drivers.
Commanding the Operating System: Shells and Terminals
Your door to the world of computer wizardry is typically the shell. Picture the shell as a translator a program that wraps around the kernel and interprets your commands. The shell takes text input (like typing ls
or cd
), then returns output, giving rise to the Command Line Interface (CLI).
Not only can you wield these powers on your own box, but if permitted you can use protocols like SSH (Secure Shell) to remotely access computers over networks.
Programming Languages and Types: Understanding Abstraction
Now that you own the terminal, you’ll need to pick a programming language. Programming languages are designed to abstract away the complexity of hardware, letting you focus on what you want to do rather than how the CPU implements low-level details.
- Interpreted Languages: Languages like Python are interpreted line by line by an interpreter. This means code runs as it’s read, offering flexibility and ease of debugging.
- Compiled Languages: Languages such as C use a compiler. It translates the entire program to machine code ahead of time, resulting in a standalone executable that the operating system can run directly.
Regardless of your language, you’ll need to wrangle data often using friendly types like numbers and characters. The way you use and label data in your code is through variables: named placeholders for reusing and referencing data.
Dynamic vs Static Typing
- Dynamically typed languages (e.g., Python) allow you to declare variables without specifying their data type. The interpreter figures it out.
- Statically typed languages (e.g., C, Java) force you to specify the type so that memory is allocated properly.
Underneath, your variables’ values are tucked away in RAM, with each variable taking up a particular memory address.
Pointers and Memory Management
Some languages let you perform low-level magic via pointers variables that store the address of another variable, empowering you to manipulate memory directly. For those who’d rather avoid such shenanigans, most modern languages provide garbage collection to automatically reclaim memory when it’s no longer in use.
Data Types, Endianness, and Data Structures
Primitive Data Types
- int: Represents integers, which may be signed (can be negative) or unsigned (only positive).
- float: Used for decimal numbers, providing a certain precision picture the scientific notation for computers.
- double: Like float, but allocates twice the memory for enhanced precision and range.
- char: A single character.
- string: A series of characters (think: "Hello, world!").
Under the hood, how characters are stored can vary: If the most significant byte is in the smallest address, it’s called big-endian; if the least significant byte comes first, it’s little-endian.
Common Data Structures
- Array (or List): Orders elements in a sequence, indexed from zero. Think of your shopping list, where each item has a spot.
- Linked List: Each item points to the next, forming a chain. Efficient for insertions and deletions.
- Stack: Last-In, First-Out (LIFO) like a stack of plates: add to the top, remove from the top.
- Queue: First-In, First-Out (FIFO) stand in line, get served in the order you arrived.
- Hash (Map/Dictionary): Stores key-value pairs for quick lookups, like a word and its definition.
- Tree: Hierarchical structure, good for tasks like sorting and searching (e.g., file directory trees).
- Graph: Nodes connected by edges useful for representing networks or relationships with complex connections.
Algorithms and Code Logic
Data needs action enter algorithms, the step-by-step recipes that turn input into output. The heart of an algorithm is the function: a block of code with a name, takes parameters (“arguments”), and (usually) returns a value.
Operators, Expressions, and Statements
In your functions, you’ll use a variety of operators to compare, calculate, and prod your data think ==
(equality), >
, <
(greater/less than). Such operations yield Boolean values true
or false
.
The result of any such operation is called an expression. Meanwhile, not all code produces a value: some instructions exist just to control flow or perform actions (these are statements).
Conditional Logic and Loops
- If statements: “If it’s raining, bring an umbrella; else, bring sunglasses.”
- Loops: While loops repeat a block of code as long as a condition is true. For loops usually iterate over items in an array or list.
Recursion and Stack Overflow
A function can even call itself, a party trick called recursion. But beware: without a proper exit condition, recursion can spiral into an infinite loop until the call stack your program’s memory for active functions runs out, resulting in a stack overflow error.
Measuring Performance: Big O Notation
To judge your algorithm’s performance, computer scientists use Big O notation to analyze:
- Time Complexity: How quickly your algorithm runs as the input size grows.
- Space Complexity: How much memory is required to execute your algorithm.
Algorithm Types
- Brute Force: Try every single option (effective… if you’re a robot with all the time in the world).
- Divide and Conquer: Split the problem, solve smaller chunks, combine results (e.g., binary search).
- Dynamic Programming: Solve complex problems by breaking them into overlapping sub-problems and reusing the results when needed (with memoization).
- Greedy Algorithms: Always opt for the best immediate choice (e.g., Dijkstra's algorithm).
- Backtracking: Explore all possible options, and undo choices when a dead end is hit (like solving a maze).
Programming Paradigms and Structures
Declarative vs. Imperative
- Declarative: Code describes what should happen, not how. Common in functional languages like Haskell.
- Imperative: Code details every step “do this, then that.” Used in procedural classics like C.
Most modern languages (Python, JavaScript, Kotlin, Swift) support multiple paradigms including object-oriented programming (OOP).
Object-Oriented Programming (OOP)
In OOP, a class defines a blueprint for objects, bundling properties (data/variables) and methods (functions). Classes can inherit behavior via inheritance, letting you write reusable, organized code.
Instantiating a class creates an object a real data instance stored in memory. When you need data that persists beyond a temporary function, it’s stored on the heap (a memory area that can grow or shrink), unlike the fleeting call stack.
Passing objects “by reference” means multiple variables can point to the same memory address, saving resources.
Threads, Parallelism, and Concurrency
Modern CPUs often split into threads, allowing code to run in virtual “cores” simultaneously (aka parallelism). Some languages let you directly take advantage of this multi-threaded capability for true simultaneous execution.
Many languages, though, only allow single-threaded execution but achieve the illusion of multitasking through concurrency (using event loops or coroutines), letting you handle many tasks without freezing your program.
The Cloud, Virtualization, and the Internet
Rarely do we code on bare metal these days. Most software runs in the cloud on virtual machines (VMs) software simulations of real hardware. VMs can split physical servers into tinier, more manageable “computers,” powering everything from web apps to global finance.
These machines communicate via the Internet Protocol (IP), each with its own unique IP address. IP addresses are matched to human friendly names using the Domain Name Service (DNS).
Establishing Connections and Security
Before transferring data, computers perform a TCP handshake. They trade packets (chunks of data), and for privacy’s sake, often wrap them in layers like SSL or TLS for encryption.
Next up, the HTTP (HyperText Transfer Protocol) provides the foundation for servers and clients to share webpages, data, and more.
Most modern apps use APIs (Application Programming Interfaces) channels that define how to request and send data, with REST being a common style where each URL corresponds to a resource or data type.
Printers: The Final Boss
After conquering all these concepts, remember: your proudest title as a computer scientist might just be “family tech support.” Especially when Grandma’s printer stops working (again). Console yourself you now understand everything inside and out… even if the printer awaits your troubleshooting superpowers.
Thanks for tuning in! Study these essentials, keep coding, and see you at the next learning quest.
Frequently Asked Questions
What are the most important concepts every beginner should know in computer science?
Beginners should focus on understanding the basics: how binary and machine code work, the function of the CPU and RAM, the difference between interpreted and compiled languages, core data types and data structures (like arrays, hash tables, and trees), the concepts of algorithms and Big O notation, and the structure of the Internet and APIs.
What is a Turing machine, and why is it important?
A Turing machine is a theoretical model that demonstrates how computers can execute algorithms. It consists of a tape divided into cells (storing 0s and 1s) and a head that can read/write, serving as a foundation for modern computation and programming languages.
How does dynamic typing differ from static typing?
Dynamic typing means you don’t need to declare the type of a variable it’s figured out at runtime (Python is an example). Static typing requires you to specify types in your code (as in C or Java), which can help catch bugs early but requires more upfront work.
What are common examples of data structures in programming?
Some of the most common data structures include arrays (lists), linked lists, stacks, queues, hash tables (maps/dictionaries), trees, and graphs. Each structure has trade-offs in speed, memory usage, and the tasks it's suited for.
What does 'Big O' notation mean in computer science?
Big O notation is a standard way to talk about the efficiency and performance (time and space complexity) of an algorithm as the problem size grows. For example, O(n) means performance scales linearly with the input size, while O(1) means performance remains constant.
What is the difference between parallelism and concurrency?
Parallelism occurs when tasks run literally at the same time (on multiple CPU cores). Concurrency refers to managing many tasks at once, often giving the illusion of true multitasking (as with an event loop), but not necessarily running at the exact same instant.
How do APIs and the Internet Protocol work together?
APIs define structured ways for clients and servers to interact, while the Internet Protocol handles addressing and sending data over the network. When a client requests data from a server via an API, the request is packaged and delivered according to IP rules, typically using protocols like HTTP/HTTPS over TCP/IP.
Why do printers always break?
Printers combine complex hardware with sometimes outdated software, network drivers, and inconsistent protocols, making them prone to errors. Understanding core computer science concepts can, however, help you diagnose and (occasionally!) fix these issues.