Full Stack Developer Roadmap 2025: The Ultimate Beginner’s Guide to Full Stack Web Development
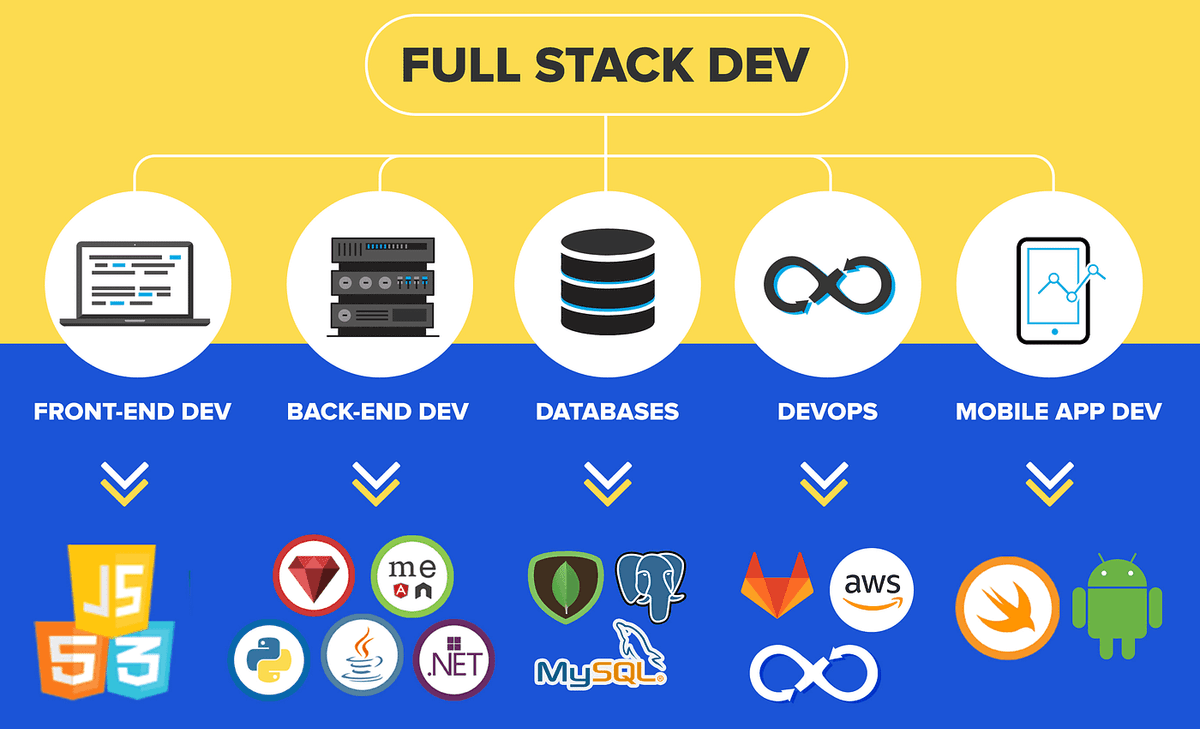
Written by Massa Medi
Web development is the best job in the world. How else could you contribute to a global digital nervous system with almost 5 billion daily active users? The internet connects us all scientists, students, entrepreneurs, meme connoisseurs in a world spanning network akin to the neurons of a superintelligent brain. Sure, it powers medical research and can drive innovation that could end poverty. But let’s be real: most of it is memes, drama, and making some sweet, sweet moolah. If you’re ready to launch your journey into web development, you’ll need to master a vast array of skills across the full stack. This guide will walk you through 101 essential concepts every aspiring web developer needs to know.
Understanding the Internet: Foundations of Web Development
The internet is essentially billions of computers linked together across the world. But how do these computers communicate? Is it like sending mail? Not quite. Instead, every computer is assigned a unique IP address thanks to the Internet Protocol Suite established on January 1, 1983 the official birthday of the internet. The Internet Protocol (IP) lets one computer identify another, while the Transmission Control Protocol (TCP) breaks data into small “packets” (like puzzle pieces) and sends them to their destination, where they’re reassembled into the original message.
Imagine these packets traveling through a maze of fiber optic cables, switches, and modems before arriving at their digital destination. It’s important to note that the internet is not the same as the World Wide Web. Think of the internet as the hardware the plumbing and the web as the software that runs on top of it.
The World Wide Web and the Power of URLs
The World Wide Web (WWW) operates as the visible layer of the internet, using the Hypertext Transfer Protocol (HTTP) to transfer information. Every resource on the web is given a Uniform Resource Locator (URL), which acts like a digital street address.
When you visit a website, you use a web browser (such as Chrome, Firefox, or Safari) to load a URL. Your browser is the client, requesting information from a server somewhere in the world. The server receives the HTTP request and sends back a response containing the HTML, CSS, images, and scripts that comprise the webpage.
Domains, DNS, and the Digital Phone Book
Every website from fireship.io to mergesociety.com needs a domain name. Domains are registered via a domain registrar accredited by ICANN, a global nonprofit responsible for managing internet namespaces.
When you navigate to a domain, your request passes through the Domain Name System (DNS), which converts that friendly address into the actual IP address of the server hosting the site. In essence, DNS functions as the “phone book” of the internet.
HTML: The Backbone of Every Web Page
The content you see on a web page is written in HyperText Markup Language (HTML). You can inspect this structure using the developer tools built into most browsers. If you want to create your own web page, use a text editor like Visual Studio Code and start building HTML documents.
An HTML document is structured as a collection of elements sections of content wrapped in opening and closing tags, like <p>
for paragraphs or <h1>
for headings. Form elements like <input>
and <select>
gather user input, while attributes (such as type="number"
or type="text"
on an input) tweak their behavior.
Anchor tags or <a>
elements are the “hypertext” in HTML, allowing you to link to new pages via URLs. All these elements are arranged in a hierarchical tree structure called the Document Object Model (DOM). Every HTML document is split into a <head>
(metainformation, invisible to users) and a <body>
(where the main content lives).
The <div>
element is frequently used to divide the page into sections. Used alone, though, all you’ll get is a bland, black and white site so how do you make your site stand out?
CSS: Designing and Styling Web Pages
The answer is Cascading Style Sheets (CSS). CSS lets you define the look and feel of your website elements. You can use:
- Inline styles via the
style
attribute (e.g.<div style="color: red;">
) - Style tags in your HTML's
<head>
section, using selectors to target elements likep { color: blue; }
- External style sheets linked from your HTML for better maintainability and code reuse
Selectors let you target as many or as few elements as necessary; you can apply CSS by tag name, by class (using className
in React or class
in HTML), or even by ID.
CSS cascades, so styles can stack and override each other based on a set of specificity rules a system that’s both elegant and quirky, like an elephant in a monocle (if you squint).
Layout and Positioning: Every element is a box with padding (internal spacing), borders, and margin (external spacing). Elements display in different ways: “block” elements span the full horizontal width; “inline” elements sit beside other inline elements. You can change an element’s position with CSS’ position
property:
- Relative: Moves an element a set distance from where it would normally be.
- Absolute: Positions an element relative to its nearest positioned ancestor.
- Fixed: Sticks an element to the same spot in the browser window, even when you scroll.
Responsive Design: Is Your Site Ready for All Screens?
Users will view your site on everything from tiny phones to massive displays. Responsive design ensures your site looks great everywhere. Media queries let you adapt your CSS based on device size or features, while powerful tools like Flexbox and CSS Grid allow elegant, efficient multi-column and multi-row layouts.
Advanced CSS: Programming with Stylesheets
While vanilla CSS isn’t technically a full programming language, it does have calc() for math operations and custom properties (variables) for reusing values. For more advanced features, you can use preprocessors like Sass, which let you write more organized, programmatic styles.
JavaScript: Making the Web Interactive
The third pillar of web development is JavaScript (JS). Technically, you don’t need JavaScript for a basic site, but if you want your pages to be more than digital brochures, JavaScript is essential. It lets you:
- Handle user interaction (clicks, inputs, animations)
- Fetch and display data without reloading the page
- Respond to events like form submissions or mouse movements
JavaScript code goes in <script>
tags or, more commonly, in separate files linked via the src
attribute. For efficiency, scripts often load with the defer
attribute so they’re executed after your HTML is fully parsed.
ES6 (EcmaScript 2015) brought major upgrades to JS, enabling developers to use let
and const
for variable declarations:
let
: Use for variables that will change laterconst
: Use for variables that stay the same
JavaScript is dynamically typed no need to declare data types. But this flexibility can sometimes cause headaches. Enter TypeScript: a superset of JavaScript that adds static typing and other developer friendly features.
Events, the DOM, and Objects in JavaScript
JavaScript shines when handling events. Every user action (a click, a keystroke, hovering) emits an event you can respond to. Grab elements with document.querySelector()
, then “listen” for events (such as click
) and provide a function for what should happen. For example, clicking a button could trigger an alert or update the page without reloading.
Data structures like arrays and especially objects (also called “dictionaries” or “hashmaps”) are core to JS. Objects can inherit properties through prototypal inheritance, allowing you to “clone” behavior through a prototype chain. JavaScript *also* supports class based syntax, but classes are mostly syntactic sugar over its prototypal system.
Modern JavaScript developers almost never touch prototypes directly. Instead, they use frontend frameworks such as React, Vue, Svelte, or Angular, which provide higher-level abstractions for building user interfaces.
Frontend Frameworks: Components and Declarative UIs
Frameworks represent your User Interface (UI) as a component tree. Each component bundles together its HTML, CSS, and JS, often looking like a custom HTML tag (<MyComponent />
). Frameworks make your code declarative, so you describe “what” the UI should look like, and the framework handles the “how” to make it so.
This approach is easier to reason about and maintain than traditional, imperative JavaScript code that directly manipulates the DOM.
BackEnd Development: Servers, Node.js, and Databases
On the other side of the web is the backend. Here, Node.js reigns supreme as the leading server-side runtime for JavaScript. Node uses the V8 engine (the same as Chrome), is single threaded, and operates on a non-blocking event loop so it’s blazing fast at handling lots of connections at once.
Node’s ecosystem is vast, thanks to the Node Package Manager (npm), which lets you download “modules” or “packages” blocks of reusable code that can be imported into your project. However, all sorts of languages can power your server, from Ruby (with Rails) to PHP (Laravel), and more.
Rendering Strategies: SSR, SPA, and SSG Explained
Full stack apps must deliver content efficiently. There are several approaches:
- Server-Side Rendering (SSR): The server generates the HTML for each request in real time. Good for content that needs to be dynamic or frequently updated. The client sends a
GET
request; the server responds with the full HTML and a status code (e.g.200
for success,404
for not found,500
for server error). - Single Page Application (SPA): The server sends a single “shell” HTML page. Routing and page changes are handled client-side by JavaScript (using frameworks like React Router). Data is fetched as needed, often in lightweight JSON format for efficiency. This approach feels “app-like” but can be tricky for search engines and social previews.
- Static Site Generation (SSG): Every page is pre-built and uploaded to the server. Content is quick to load and easy for bots and social media scrapers to understand. Modern frameworks “hydrate” (activate interactive JS on) these pages postload for SPA like experiences.
Tools like Lighthouse help developers optimize websites for performance metrics such as First Contentful Paint (FCP) and Time to Interactive (TTI).
Full Stack Frameworks, Build Tools, and Deployment
Full Stack frameworks (like Next.js, Ruby on Rails, or Laravel) abstract away tedious configurations and provide battle tested solutions for routing, data fetching, and more.
Module bundlers like Webpack and Vite assemble all your JavaScript, CSS, and assets for efficient delivery. Linters like ESLint ensure your code style stays clean and consistent.
When it’s time to deploy, modern applications often use cloud providers like AWS, and are containerized using Docker for scalable, repeatable setups. Platform as a Service (PaaS) options take care of infrastructure (for a price), or you can go the Web3 way and host on blockchains for true decentralization.
Beyond 101: The Truth About Mastery in Web Development
If all of this feels overwhelming, don’t fret! Nearly every developer, no matter their experience, relies on Google to solve unexpected problems. The field moves fast and learning never stops. Congratulations you’re now equipped with the basics of Web Development 101! Get out there, break stuff, build stuff, and most importantly, have fun.
Frequently Asked Questions
What is web development and why is it important?
Web development is the process of building and maintaining websites and web applications. It encompasses everything from creating simple static landing pages to complex, database-driven sites and dynamic web apps. Web development powers the modern connected world, making information, services, and communication accessible on a global scale.
What are the main core languages used in web development?
The three foundational languages for web development are HTML (structure and content), CSS (styling and layout), and JavaScript (interactivity and logic). Most modern full stack development also includes knowledge of backend languages (like Node.js, Python, Ruby, or PHP) and database query languages (like SQL).
How do websites become accessible via domain names?
When you type a domain name into your browser, the Domain Name System (DNS) translates that domain into an IP address pointing to a server. This allows your browser to send requests to and receive responses from the correct web server hosting the website.
What is the difference between the internet and the World Wide Web?
The internet refers to the massive network of interconnected computers and data cables worldwide. The World Wide Web is a system of interlinked documents (web pages) and resources, accessed via the internet with protocols like HTTP and HTTPS.
What are the most common web development frameworks?
Popular frontend frameworks include React, Vue.js, Angular, and Svelte. On the backend, frameworks like Node.js (with Express), Ruby on Rails, Laravel (for PHP), and Django (for Python) are widely used. Full stack frameworks such as Next.js combine both frontend and backend capabilities.
How can I make my website responsive and mobile friendly?
Use responsive design principles, employing techniques such as CSS media queries, flexbox, and CSS Grid to ensure your layout adapts smoothly to various device sizes. Testing across multiple devices and screen resolutions is also crucial.
What tools do web developers use for testing and deployment?
Developers use tools like Lighthouse for performance audits, ESLint for code quality, and build tools/module bundlers like Webpack or Vite to prepare code for production. Deployment relies on cloud providers (AWS, Azure, GCP), platforms like Vercel or Netlify, and containerization solutions like Docker.
How do websites stay secure?
Websites implement authentication (verifying user identities), authorization (granting permissions), HTTPS encryption, and many other best practices such as input validation, regular software updates, and using secure, well tested frameworks.
What is Full Stack development?
Full stack web development means working on both the client side (front end) and server side (back end) of web applications, often including database management and deployment. Full stack developers have a broad understanding of how every layer of a web application works.