Docker 101: Mastering Modern Software Delivery with Containers
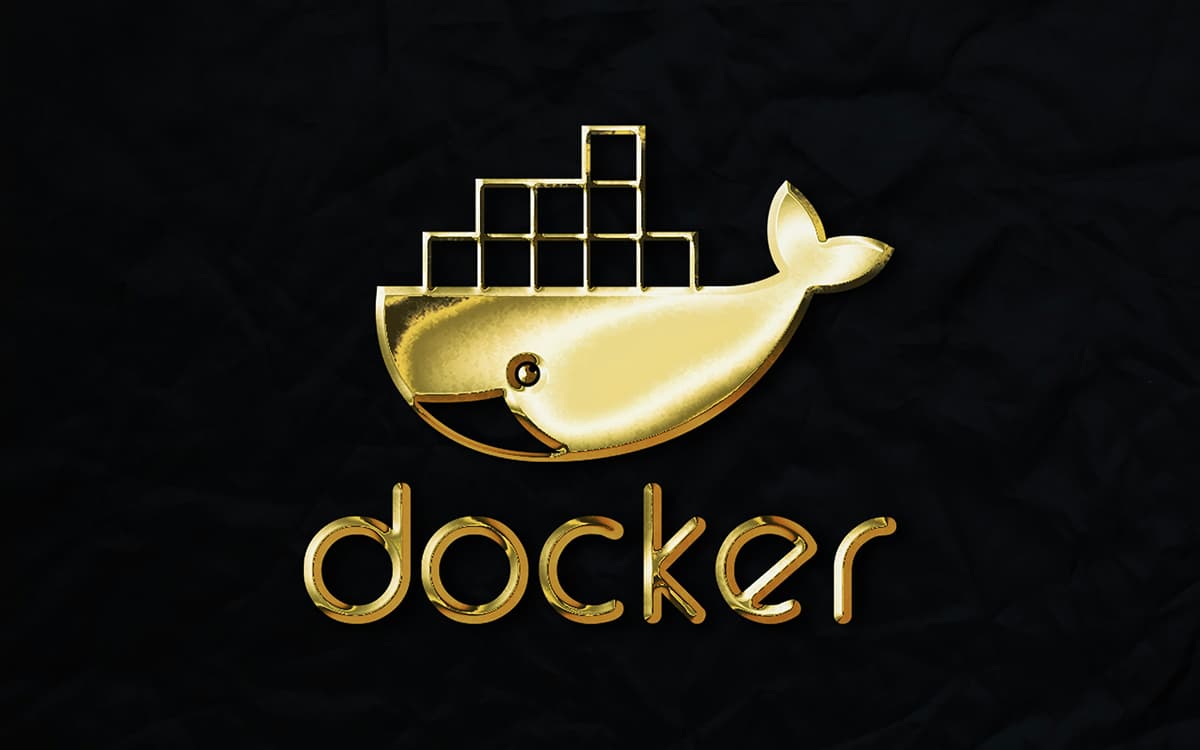
Written by Massa Medi
Welcome to Docker 101 your launchpad into the world of containerization and modern software deployment. If you plan to ship software that actually works out there in the wild, understanding containerization isn't just a buzzword it's a total game changer. Whether you're grinding away on your laptop or deploying applications on a global scale, Docker can help you banish two classic developer headaches: "It works on my machine" and "This architecture doesn't scale".
Why Containerization Matters
Let’s face it software used to be a physical thing. In the old days (think: big box stores, software on CDs), you’d actually install applications by hand onto your desktop. Today, almost all software is shipped via the internet, leveraging the intricate magic of networking. But this new convenience brings new infrastructure challenges, especially at scale.
Imagine streaming a YouTube video. Your computer acts as the client, while billions of others access those same cat videos from remote computers called servers. As more users pile in, strange issues emerge CPUs max out, disks grind under high I/O, bandwidth gets gobbled up, and databases become unwieldy. To make matters worse, your code might introduce bugs like race conditions or memory leaks that eventually cripple your entire server.
Scaling 101: The Vertical vs. Horizontal Dilemma
So, how do we scale? There are two core strategies:
- Vertical scaling: Make your server more powerful add more RAM or CPUs. Eventually, though, you’ll hit a hardware or budget ceiling.
- Horizontal scaling: Spread your work across many smaller servers, often breaking your app into microservices that run independently. This method is super effective but incredibly tricky with traditional (bare metal) hardware.
To stretch the limits further, engineers turned to virtual machines (VMs). With a Hypervisor, you can run multiple operating systems isolated on a single box. VMs are great, but they come with fixed resource allocation and don't offer flexibility for modern cloud apps.
Enter Docker: Flexible, Dynamic, and Developer Friendly
Here’s where Docker takes the spotlight (and, in this case, kindly sponsors us). Docker applications share the same host operating system kernel, dynamically using resources as needed. Under the hood, the Docker engine runs a persistent background process (a daemon) that brings OS level virtualization to the masses.
With Docker Desktop, getting started is a breeze. Developers can easily sandbox their apps without reconfiguring their entire development environment.
Docker’s Three Step Magic: From Blueprint to Container
- Start with a Dockerfile: This is your configuration blueprint a set of instructions detailing how your app's environment should be built.
- Build a Docker image: Using the Dockerfile, Docker assembles an image containing the OS, your code, and every dependency needed to run the app. This image can be shared and uploaded to public repositories like Docker Hub.
- Run a container: An image alone is just potential. By running it, you create a live, isolated container an instance of your app scaled to your needs, and ready to work anywhere.
Containers, by design, are stateless if they shut down, any internal data is lost. But that’s what makes them so portable; they can run on virtually any cloud service with no vendor lock in. Truly, the world is your oyster.
Hands on: Building a Dockerfile Step by Step
Ready to see Docker in action? Let's build a Dockerfilethe master recipe for your application container.
- FROM: The foundational instruction. This typically points to a base image (like a Linux distro) and may specify a version tag (e.g.,
:latest
). - WORKDIR: Creates a directory inside your image and sets it as the working directory for all next commands.
- RUN: Execute commands (for example, installing dependencies using a package manager), just like you would in a terminal.
- USER: By default, you’re root. But for better security, create and switch to a non root user.
- COPY: Moves your application code from your local machine into the image.
Take a breather you’re already halfway there!
- ENV: Set environment variables (like API keys) that your app needs.
- EXPOSE: Specify which ports should be available for outside traffic (for example, port 80 for a web server).
- CMD: This is the command that's run when your container starts typically the command to launch your server. Only one
CMD
per container, though you can also useENTRYPOINT
for additional flexibility.
For bonus points, you might add:
- LABEL: Include metadata about your image.
- HEALTHCHECK: Define steps Docker should use to confirm your container is healthy and running as expected.
- VOLUME: Attach persistent storage or share files between multiple containers using volumes.
That’s a complete Dockerfile! Now, what next?
Building and Managing Docker Images
After installing Docker Desktop, you gain access to the Docker CLI. Open your terminal and type docker help
for a list of commands. To turn your Dockerfile into an image, use docker build
. The t
flag lets you tag your image with a human friendly name.
As Docker builds the image, you’ll notice it processes layers. Each layer is identified by a unique SHA 256 hash. If you tweak your Dockerfile, Docker cleverly caches unchanged layers making future builds much faster and more efficient.
Don’t want to include certain files (like node_modules
or secret config files) in your image? Just add them to your .dockerignore file similar to .gitignore
to keep your image lean and secure.
Jump into Docker Desktop and check out your shiny new image! Here, you’ll get a layer by layer breakdown. With Docker Scout, you can even scan for security issues in each layer. Docker Scout works by extracting the image’s software bill of materials and cross referencing vulnerabilities with security databases, providing severity ratings to help you prioritize any fixes.
Running, Stopping, and Managing Containers
The moment of truth! In Docker Desktop, press Run to fire up your container (behind the scenes, this runs the docker run
command). You’ll be able to access your app say, a web server on your computer’s localhost.
Inside Docker Desktop’s UI, you can see all running (and stopped) containers mirroring what you’d get using docker ps
or related commands in the terminal. Click any container to:
- Inspect its logs for troubleshooting.
- Browse its internal file system.
- Execute commands directly inside the running environment.
When you’re done, gracefully stop your container with docker stop
or force it down with docker kill
. Remove stopped containers with docker rm
to keep your environment tidy.
Taking Containers to the Cloud (And Beyond)
Ready for production? Use docker push
to upload your image to a remote registry for deployment on cloud platforms like AWS Elastic Container Service or Google Cloud Run (which even supports serverless deployments!).
Want to use an image someone else built? docker pull
lets you download it, run it, and avoid all the messy environment setup no changes needed on your machine!
Congratulations! Whether you're deploying personal web apps or building the next unicorn startup, you’re now officially a Docker expert. Go ahead, print out your Docker certificate and impress your next interviewer they probably need Docker help, too.
Beyond the Basics: Docker Compose and Orchestration
Docker’s real superpower shows when building multi service apps. Enter Docker Compose a tool to manage multi container scenarios. Define your stack (frontend, backend, databases, etc.) in a single docker compose.yml
file. Then, launch everything at once with docker compose up
, or take it down just as fast with docker compose down
.
One server to rule them all? That'll work up to a point. But to truly scale to massive, global systems, you’ll eventually need an orchestration tool the reigning king is Kubernetes.
Kubernetes: The Heavyweight Orchestrator
Kubernetes (born at Google, inspired by the Borg system) seamlessly deploys and manages containers at scale. Here’s a high level view:
- Control Plane: Exposes an API to manage your cluster.
- Cluster: Composed of nodes (servers), each running a Kubelet and one or more pods.
- Pod: The smallest deployable unit, often housing one or more containers.
What makes Kubernetes so powerful? You define your application's desired state, and Kubernetes automagically adjusts the environment: scaling up, scaling down, and providing self healing if something goes sideways. But let’s be honest unless you're running a huge, complex, high traffic service, you probably don’t need Kubernetes just yet.
(Pro tip: If you do, Docker Desktop supports Kubernetes extensions for debugging pods right from your desktop.)
Wrapping Up: Your First Steps in Containerization Mastery
Congratulations on making it through 101 concepts of containerization! Docker has transformed how we develop, test, and deploy applications, taking us from "it works on my machine" to "it works everywhere". Big shoutout to Docker for powering this learning journey, and hats off to you for becoming a certified Docker expert!
Now go forth, print that certificate, and show off your new container skills at your next job interview or, better yet, on your next big project. The future of scalable, portable, and efficient software delivery is in your hands.
Recommended Articles
Tech
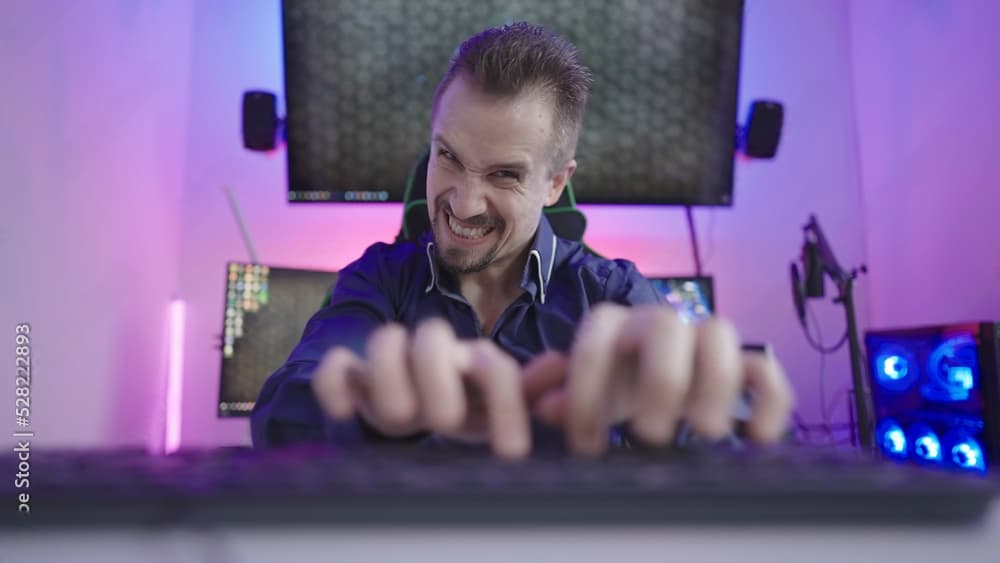
The Essential Guide to Computer Components: Understanding the Heart and Brain of Your PC
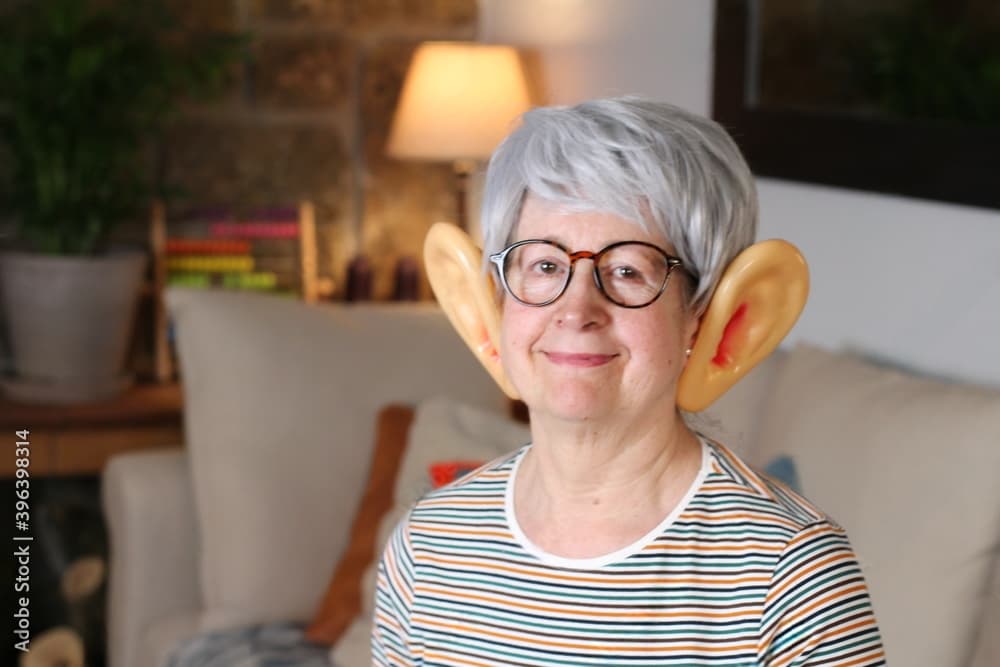
Google’s Antitrust Battles, AI Shenanigans, Stretchy Computers & More: Your Wild, Weird Week in Tech
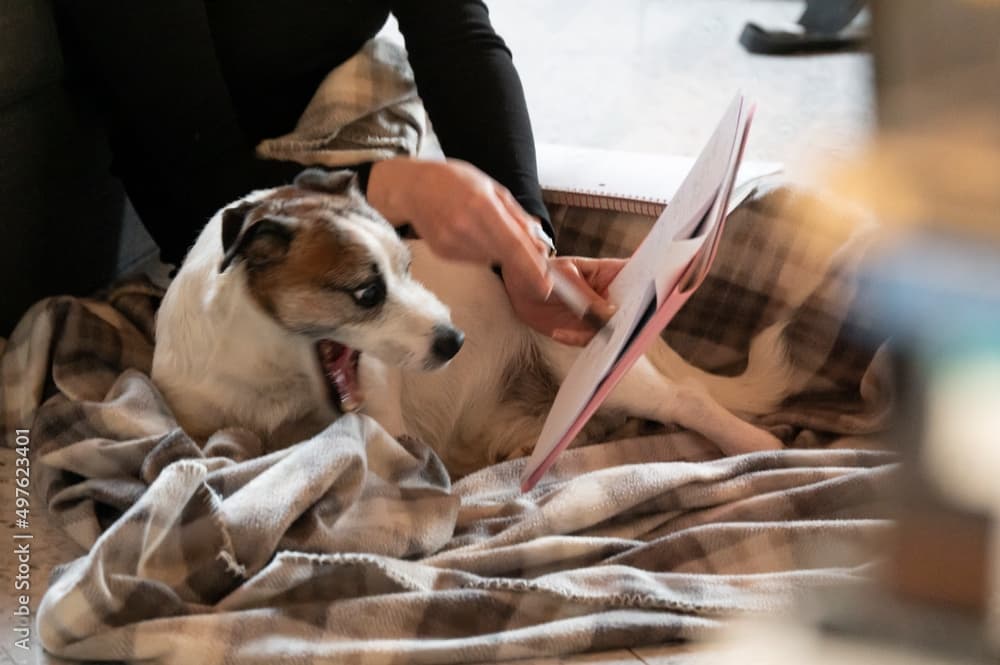
The Ultimate Guide to Major Operating Systems: From Windows to Unix and Beyond
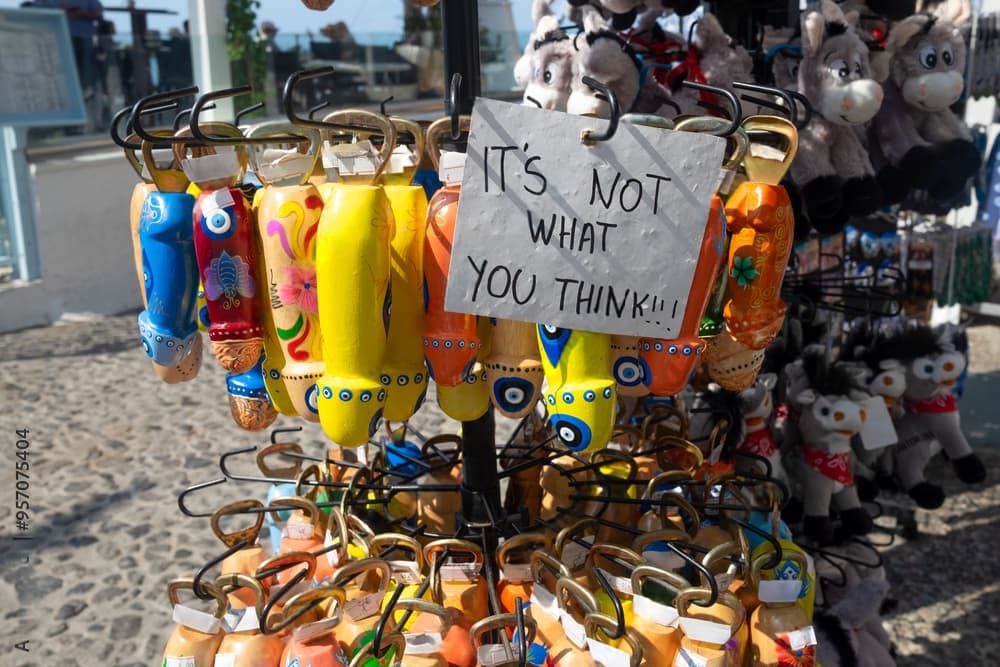
Palantir: How a Silicon Valley Unicorn Rewrote the Rules on Tech, Data, and Defense
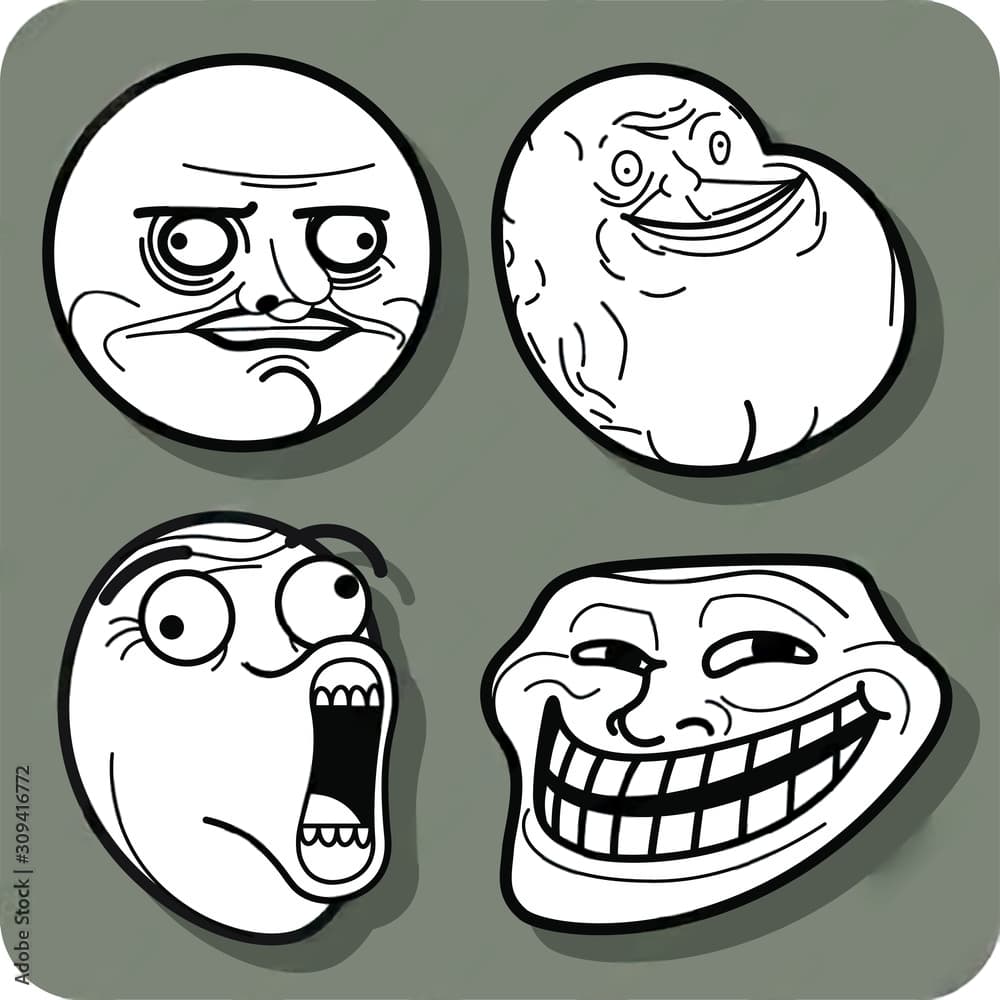
The Secret Magic of Wi-Fi: How Invisible Waves Power Your Internet Obsession
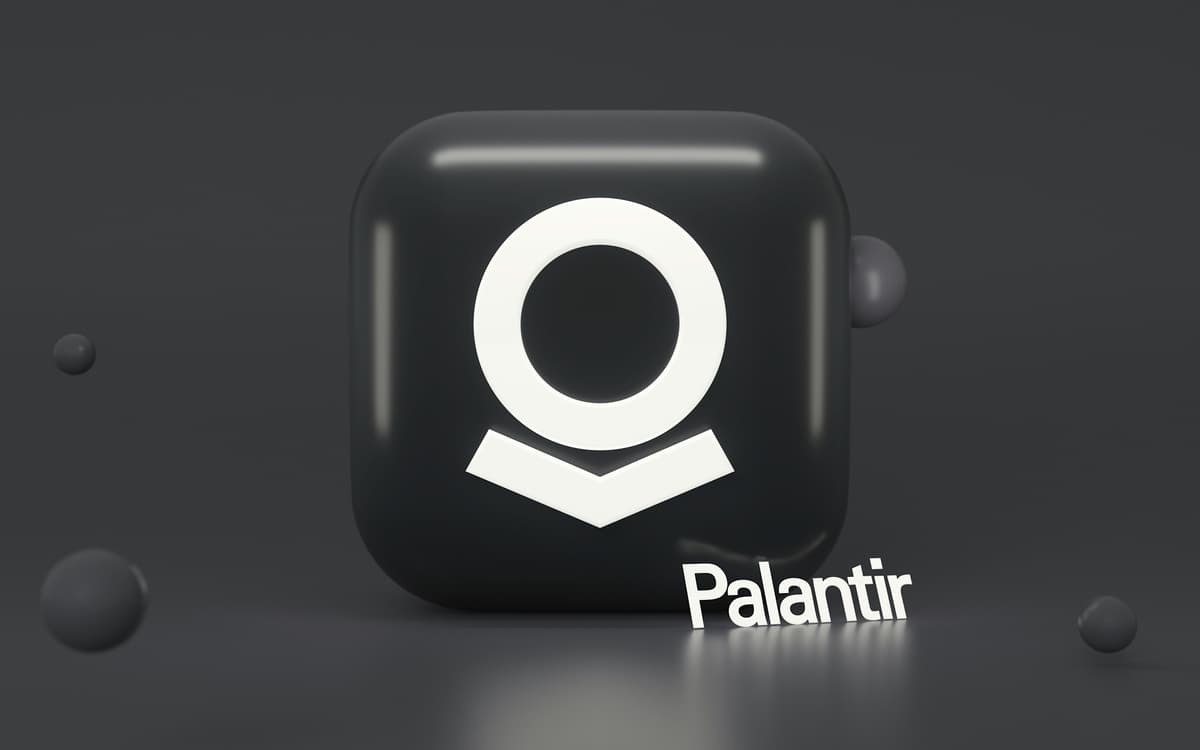
Palantir: The Shadow Tech Giant Redefining Power, Privacy, and America’s Future
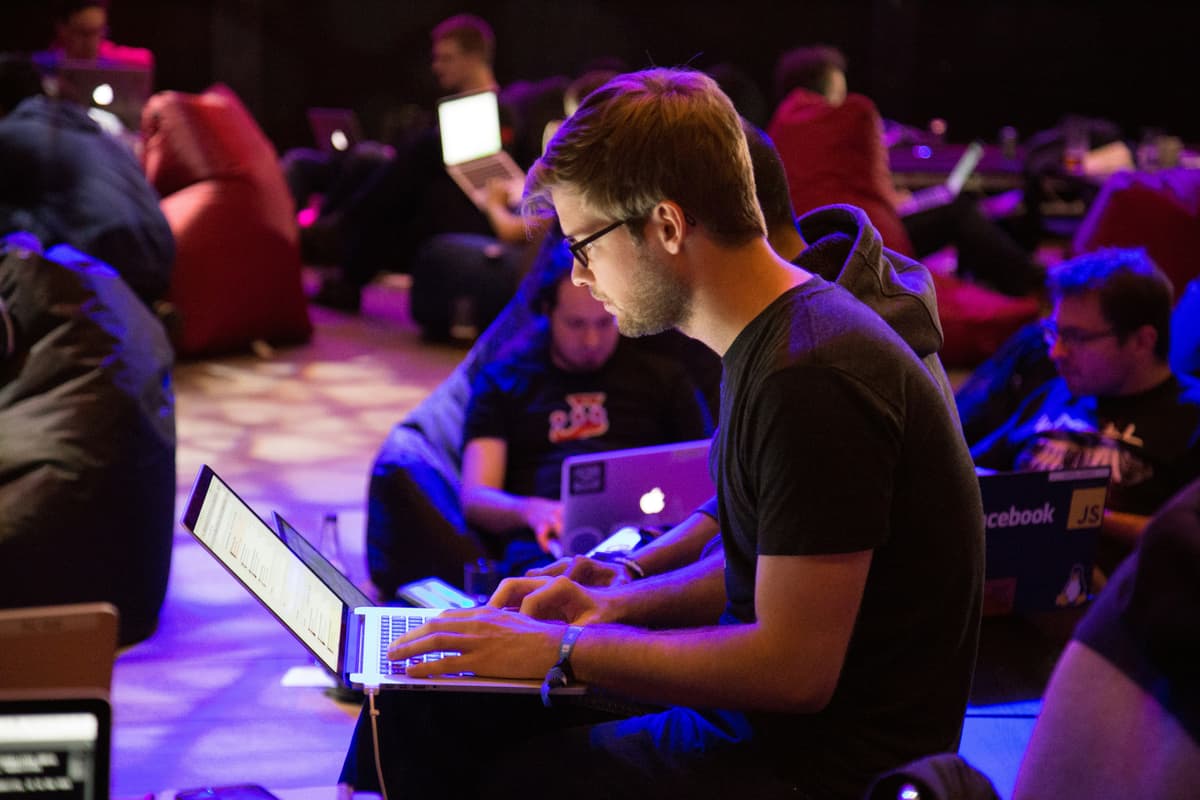
Inside Tech’s Wild Subcultures: From Devfluencers to Codepreneurs—A Candid Exposé
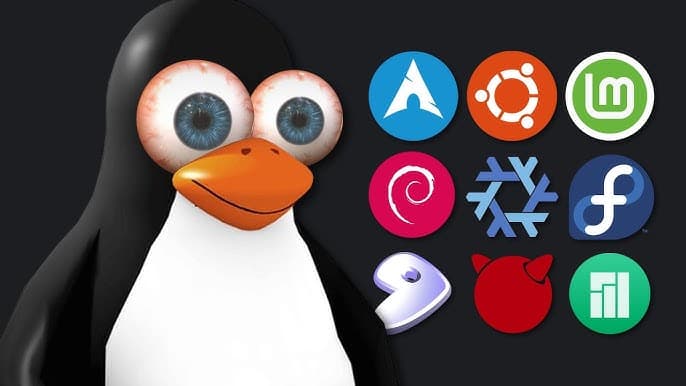
The Life Cycle of a Linux User: From Awareness to Enlightenment (and Everything in Between)
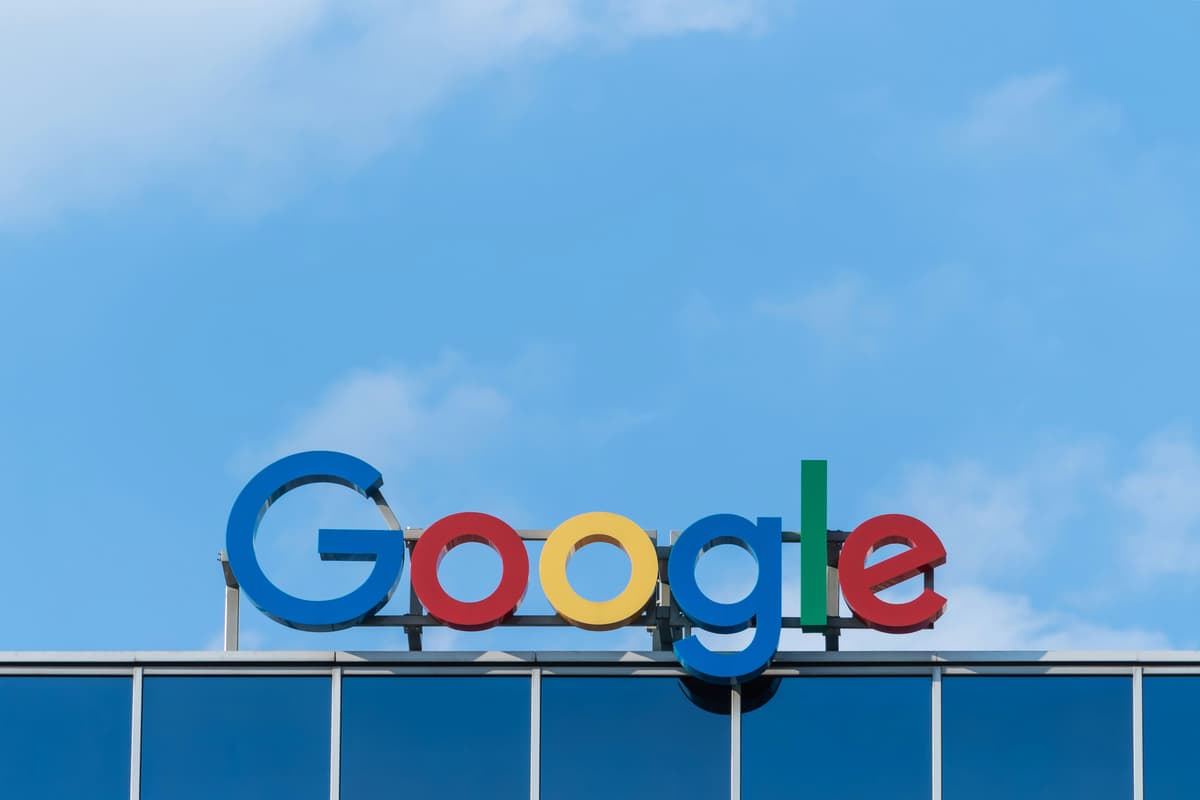
How to apply for a job at Google
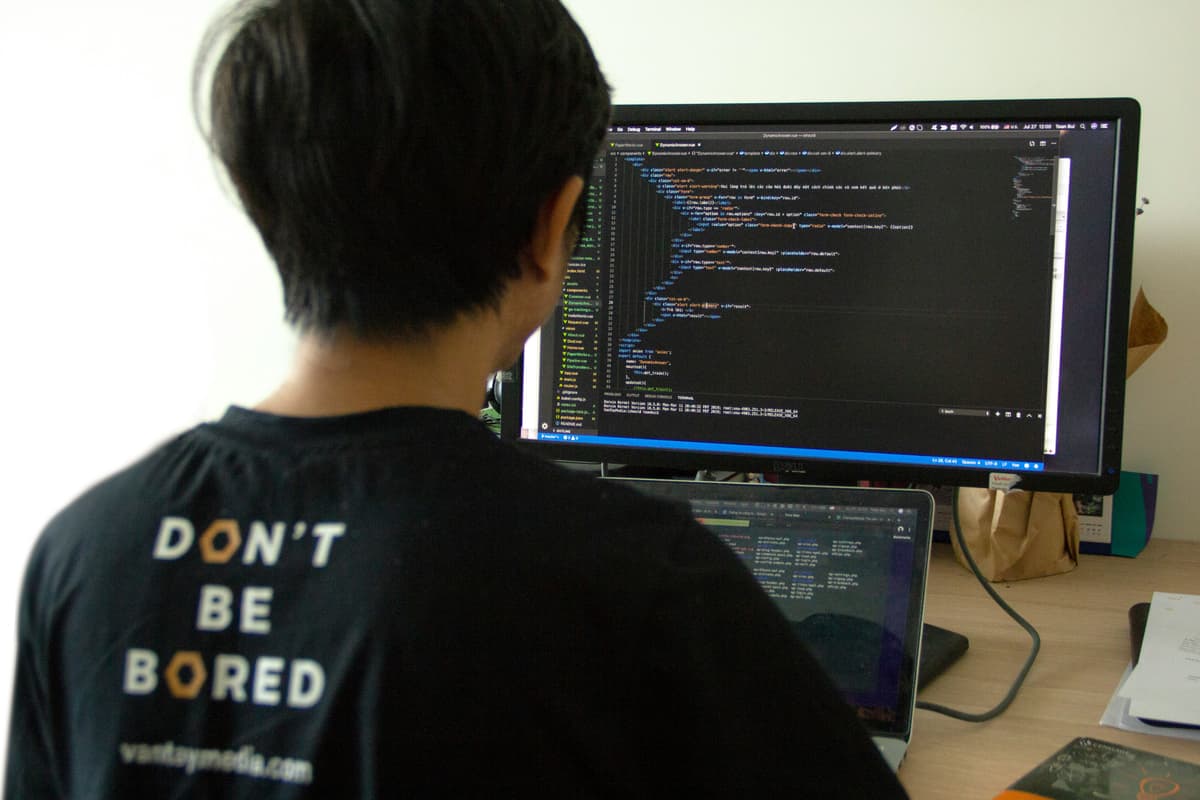
40 Programming Projects That Will Make You a Better Developer
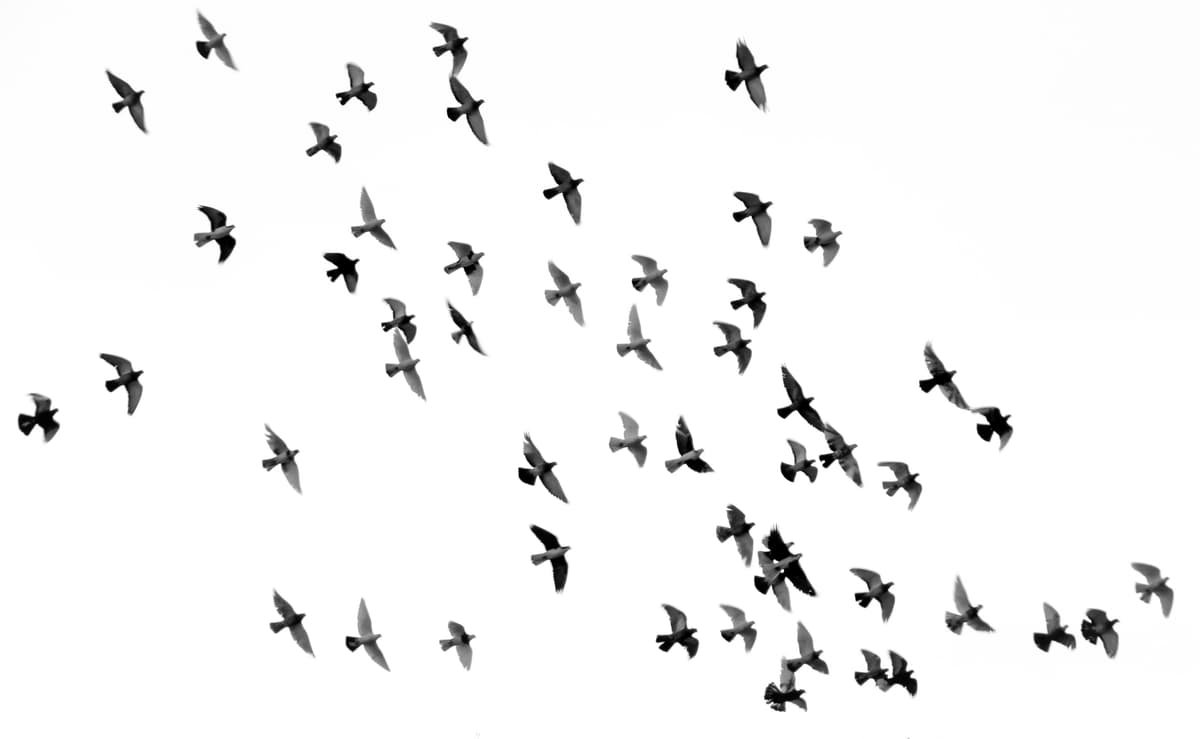
Bird Flu’s Shocking Spread: How H5N1 Is Upending America’s Farms—and the World Isn’t Ready
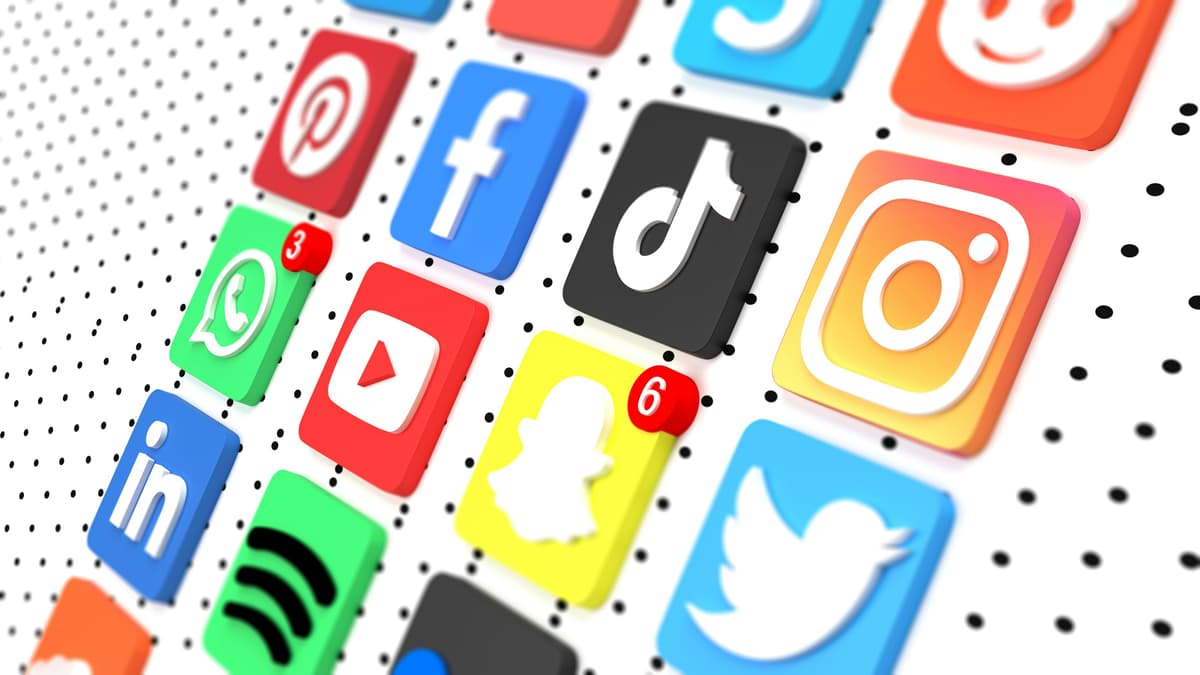
AI-Powered Bots Offend Reddit, Infiltrate Communities, and Power High-Tech Scams: What You Need To Know in 2025
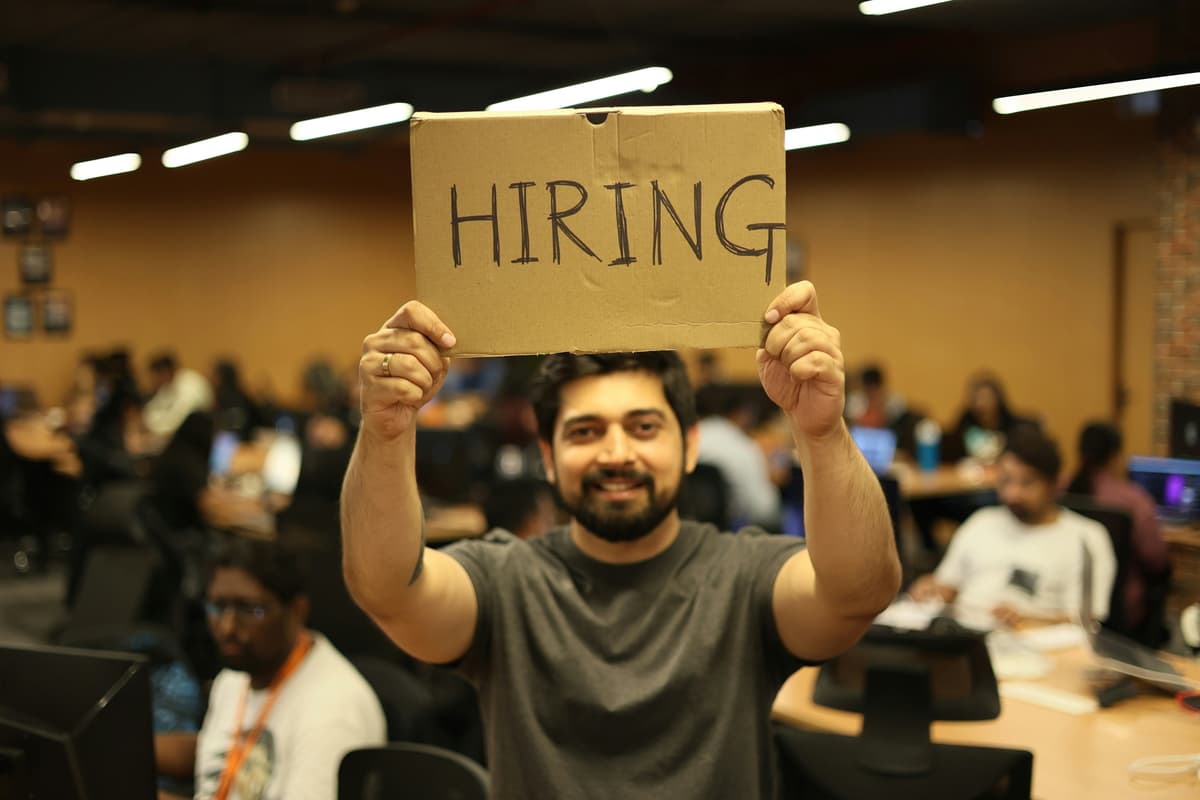
Tech Jobs in 2025: Will the U.S. Tech Job Market Bounce Back as AI Takes Hold?
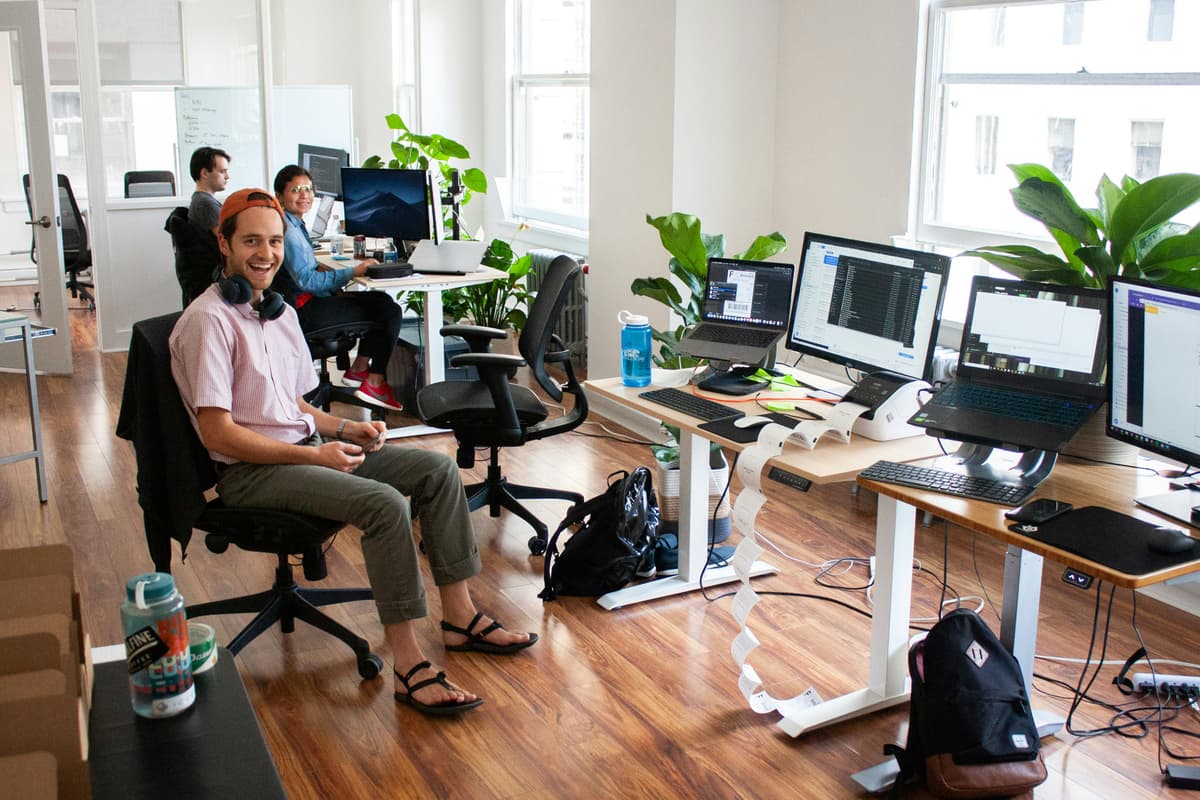
Tech Jobs in Freefall: Why Top Companies Are Slashing Job Postings Despite Record Profits
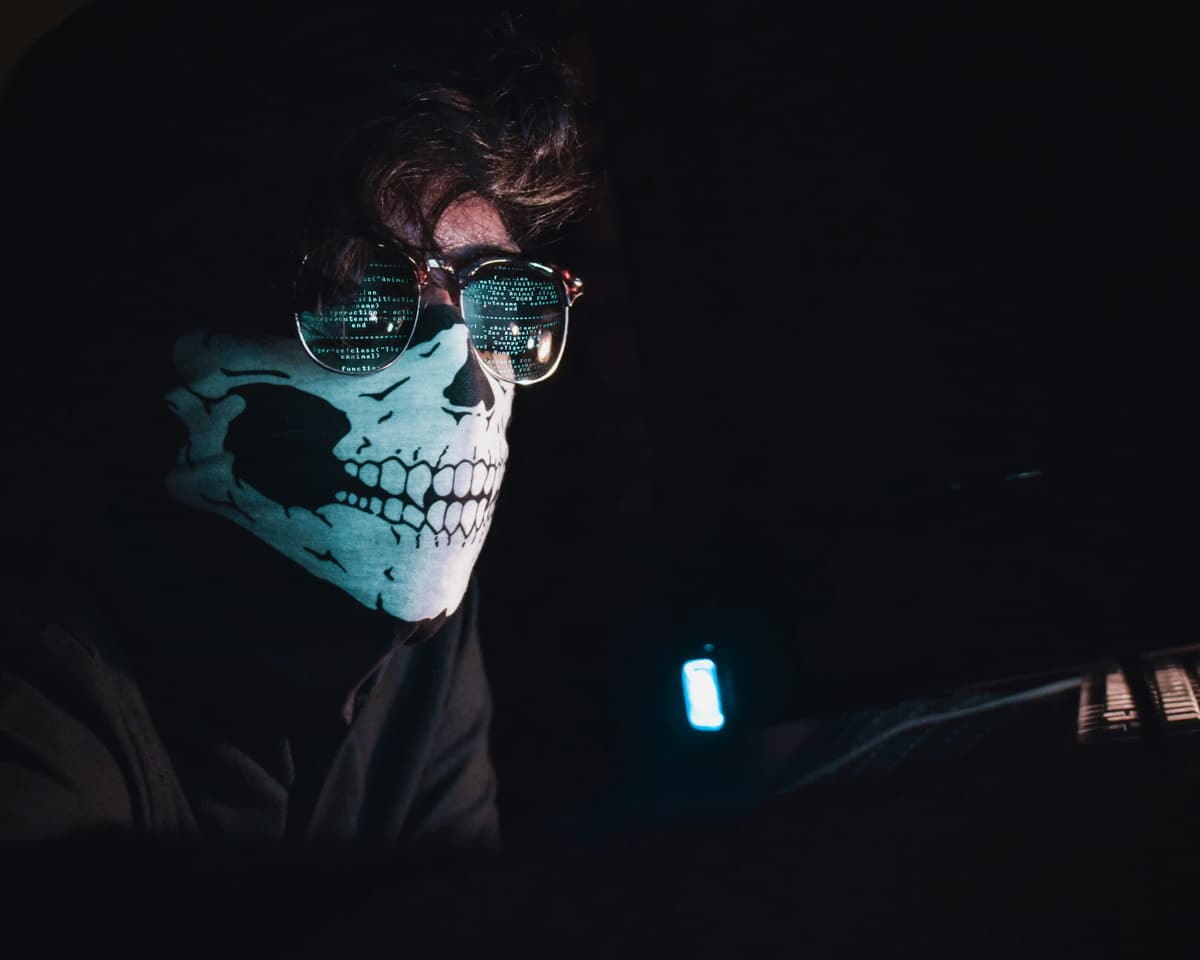
The Greatest Hack in History
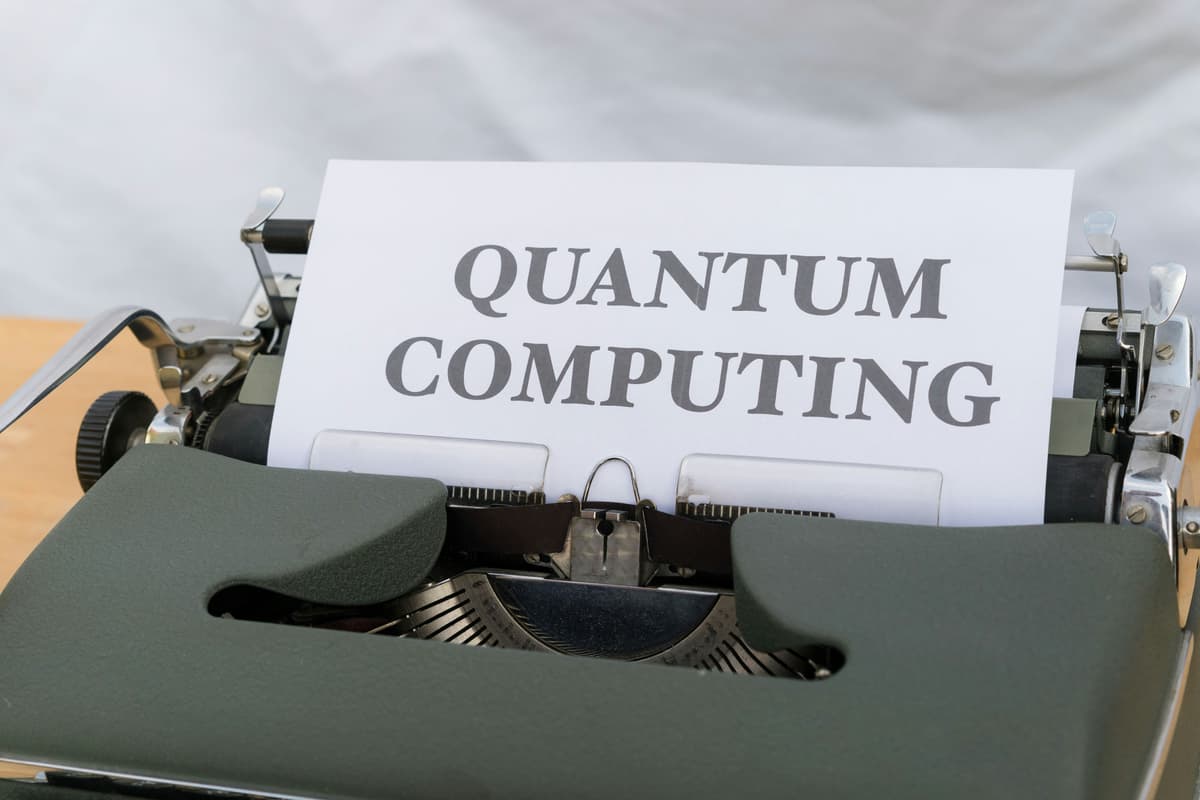
But what is quantum computing? (Grover's Algorithm)
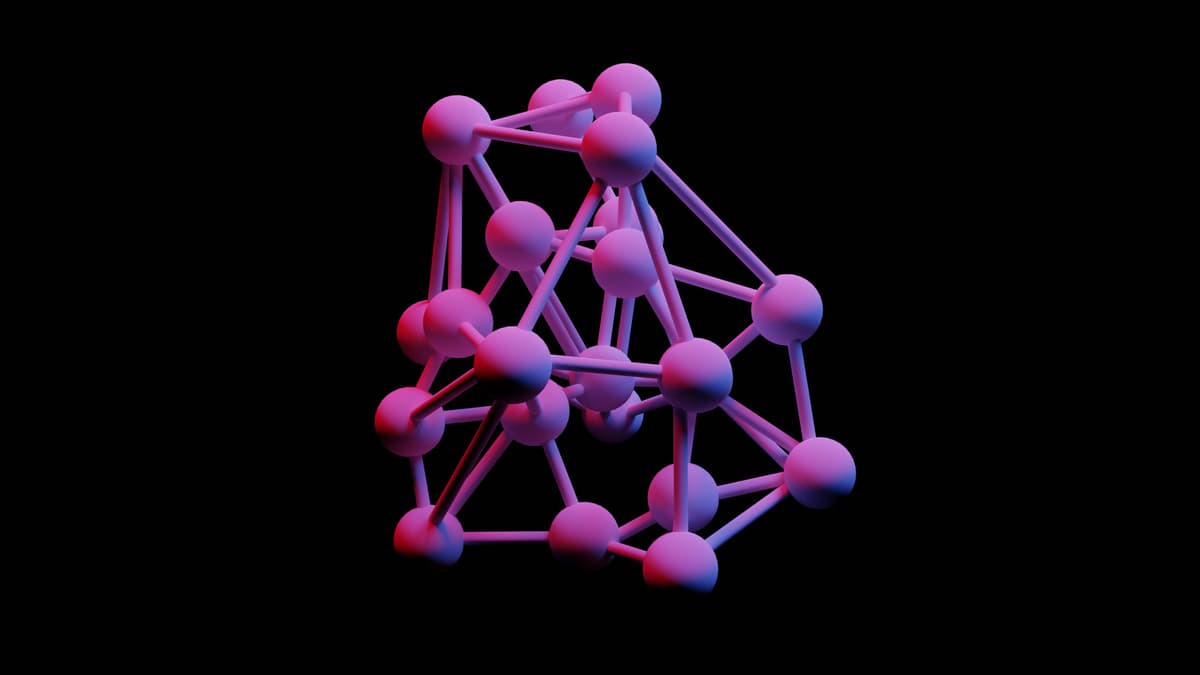
But what is a neural network? | Deep learning
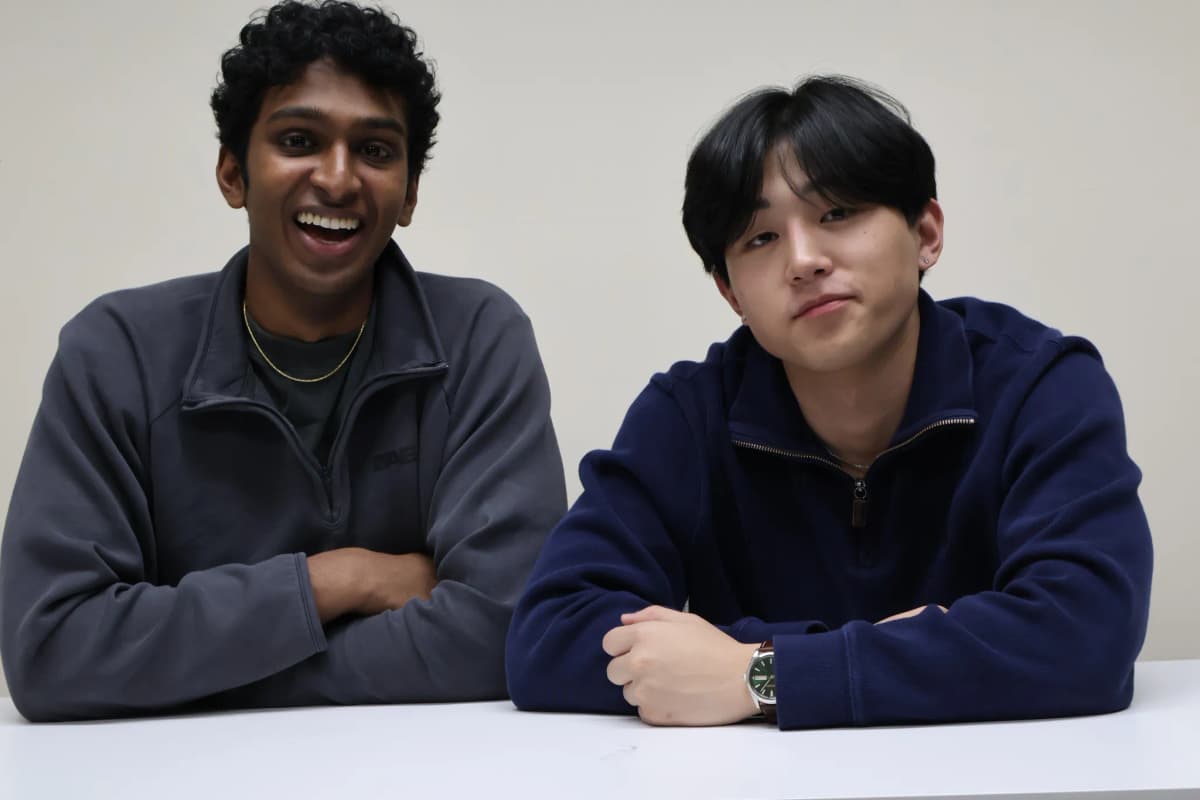
The Rise and Fall of Roy Lee: What His Story Means for Tech Recruiting (And Why Whiteboard Interviews Aren’t the Real Problem)
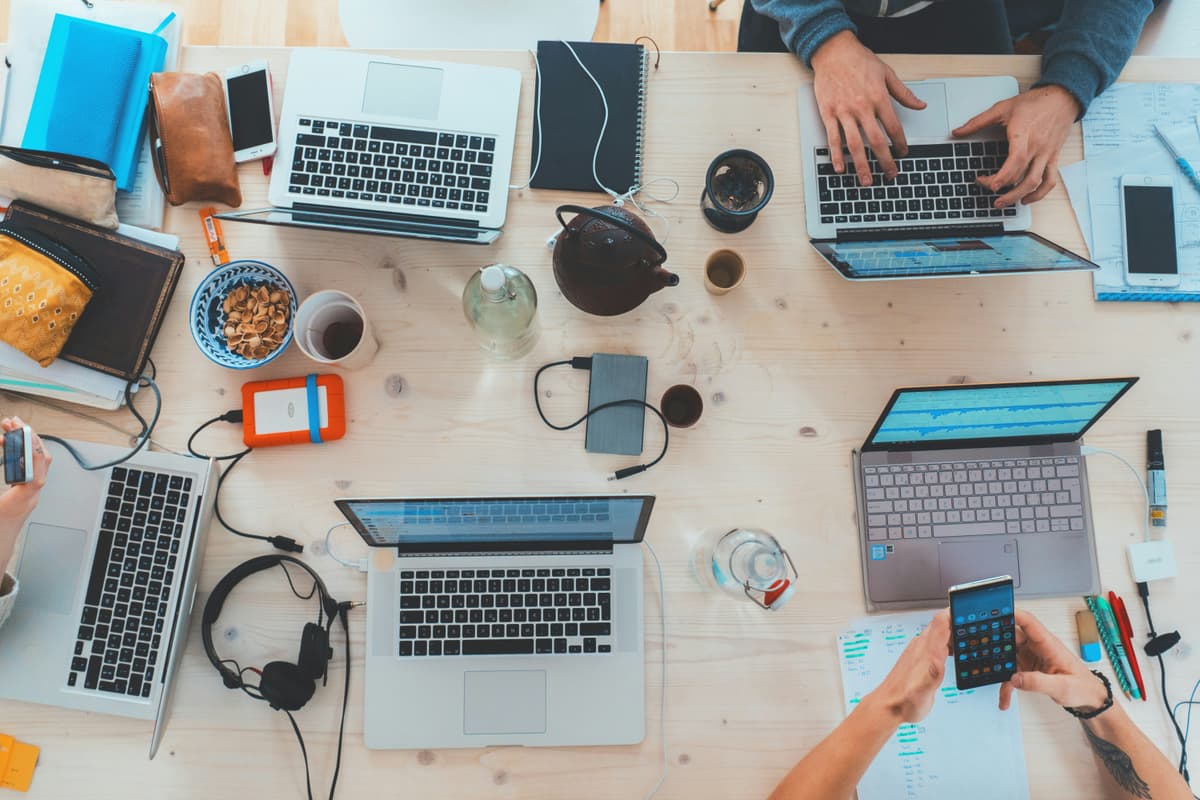
What It's Really Like to Study Computer Science: Reality of CS Majors
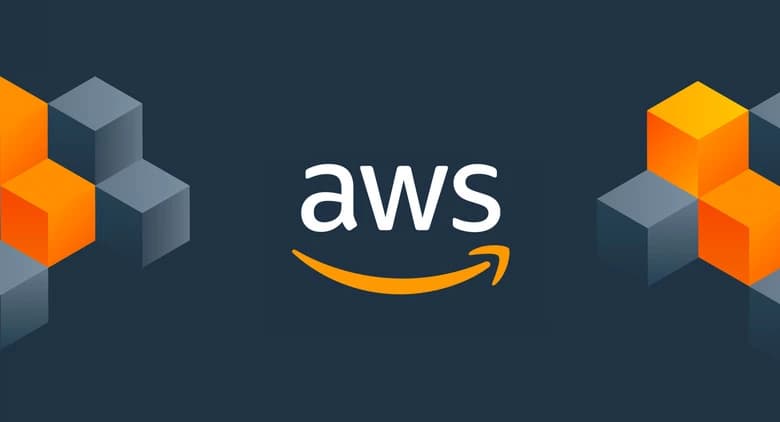
Top 50+ AWS Services Explained: What They Do and How They Power the Cloud
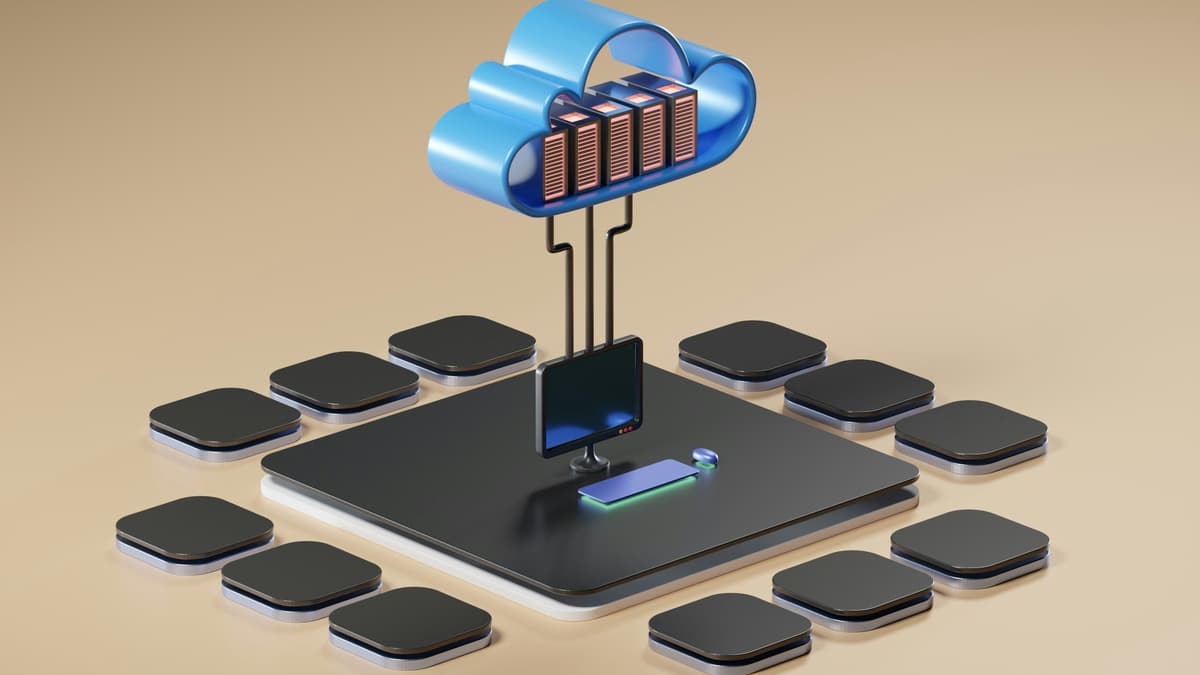
Top 50+ AWS Services Explained: What They Do and How They Power the Cloud
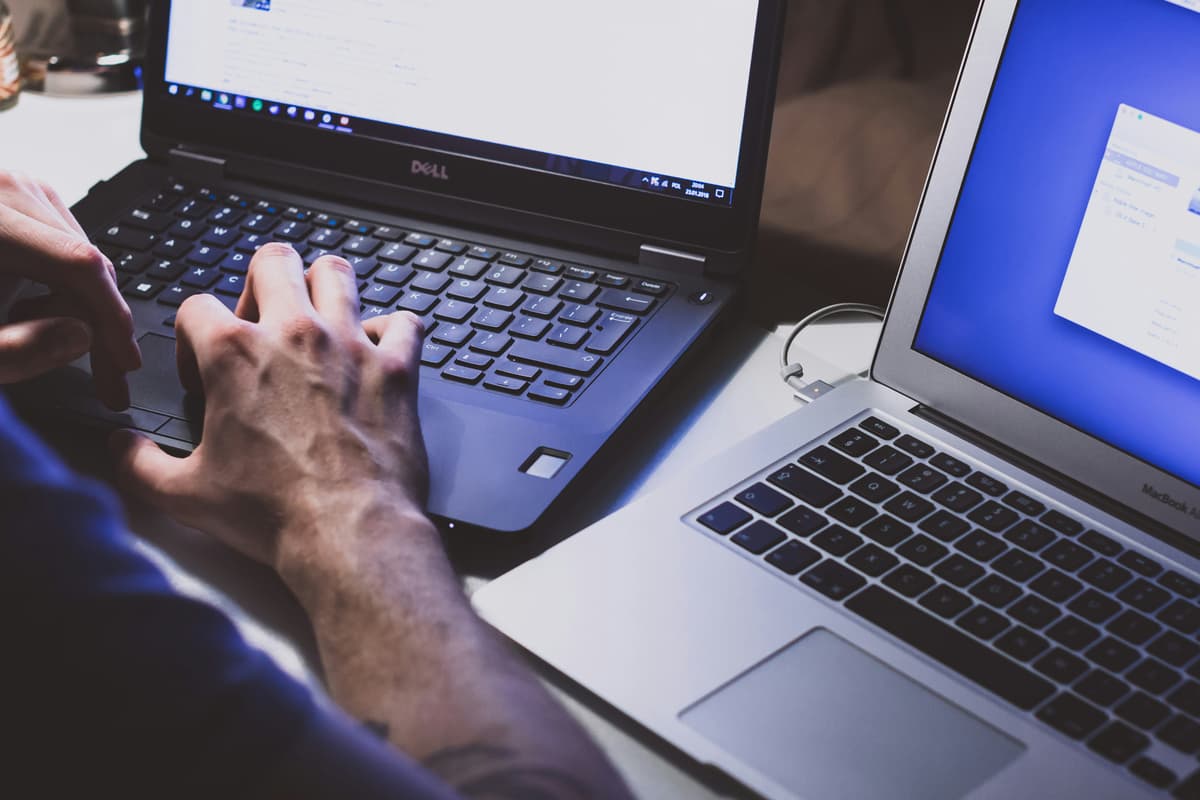