Python Libraries: The Top 40 Essential Python Libraries You Can't Ignore in 2025 (With Real Examples & Pro Tips)
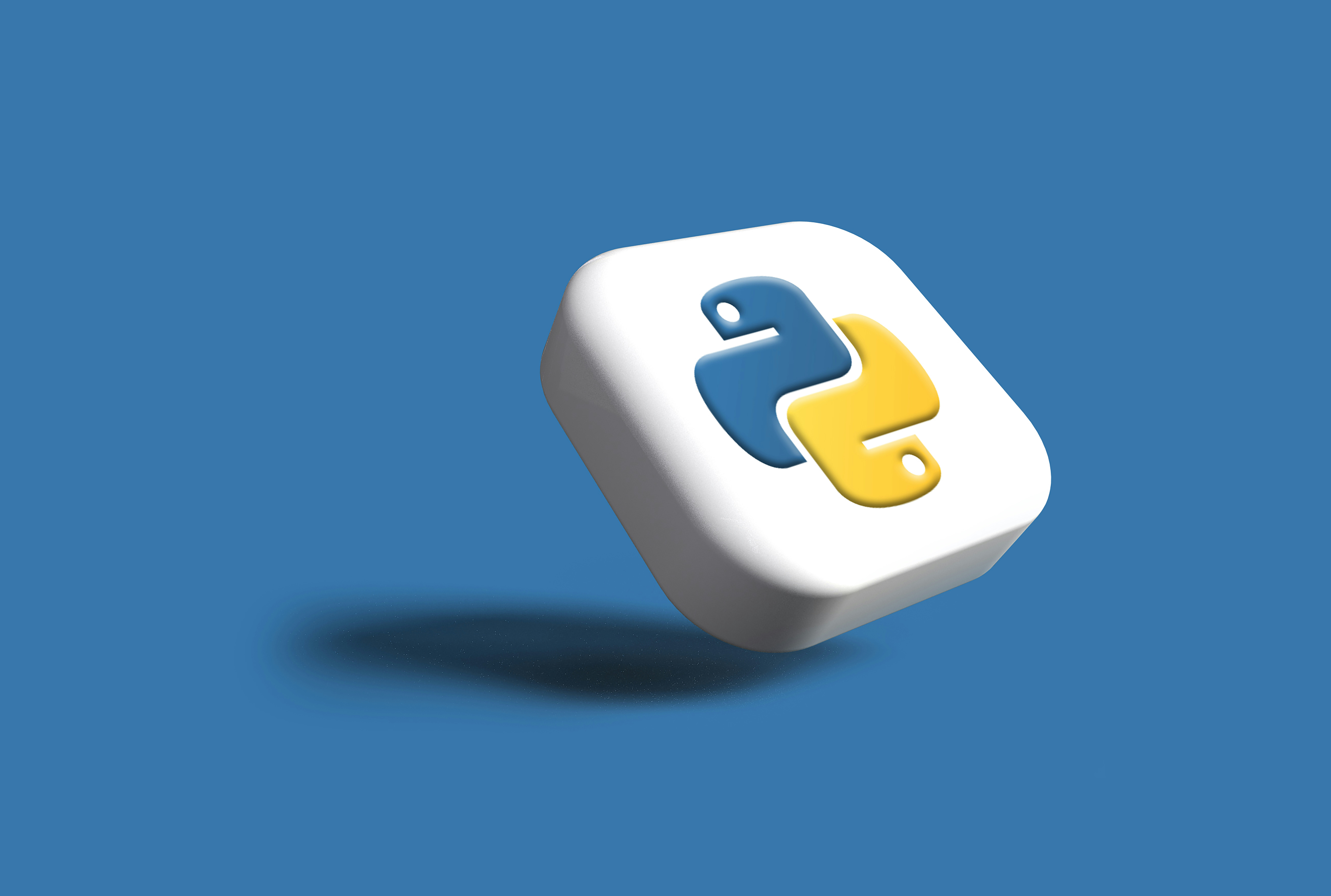
What if I told you that mastering a handful of Python libraries could 10x your productivity, open doors to incredible projects, and make you look like the smartest coder in the room? Most people dabble with a few Python packages—but almost nobody knows which libraries actually run the coding world in 2025. Today, I’m pulling back the curtain and giving you the ultimate arsenal of Python’s 40 most powerful libraries. Miss one, and you’re leaving insane opportunities on the table—guaranteed.
Natural Language Processing Libraries in Python: NLTK, Gensim & FlashText
Why NLP Isn’t Optional Anymore
Here’s what nobody talks about: Every hot field—AI chatbots, search engines, digital assistants, data mining—runs on natural language processing (NLP). But with over 250 Python libraries, choosing the wrong one will waste months of your life.
Natural Language Toolkit (NLTK): Your Language Hacking Toolkit
- Tokenization: Turns walls of text into bite-sized words or sentences. Run a line like
tokenize("New York Muffins are tasty")
and get a neat list: ["New", "York", "Muffins", "are", "tasty"]. - POS Tagging: Instantly tag every word, so “John” gets labeled as
NNP
(proper noun). - Stemming: Chop words to their roots—“maximum” becomes “maxim,” “presumably” becomes “presume.” Beware: different stemming algorithms = different results, so always double check the output.
When you stack these features together, you’re not just analyzing boring text: you’re building chatbots, stock sentiment analyzers, and beyond. NLTK is a classic for a reason.
Gensim: Memory-Efficient, Multi-Core NLP for Pros
While NLTK shines for beginners, Gensim targets those hungry for large-scale text analytics and vector magic.
- No Memory Problems: Stream huge data easily.
- Topic Modeling Power: Jump into hot algorithms like LSA, LDA, and Random Projections.
- Docs & Tutorials: Their Jupyter notebook guides are some of the best out there.
FlashText: The Secret Weapon for Fast Text Search
“Why fight regex when FlashText will lap it on big data?” That’s not hyperbole—it’s benchmarked fact. If you need speed for finding, replacing, or deleting keywords in massive texts, FlashText destroys regex after 500+ words. Just note: no special character searches here.
“Success isn’t about working harder—it’s about using tools everyone else ignores.”
Computer Vision: OpenCV, SimpleCV, & the Underdogs
Why Computer Vision Libraries Are Exploding in 2025
Image recognition, object tracking, even facial makeup applications—CV is everywhere. But you’ll only crush it if you pick smart.
OpenCV: The Godfather of Computer Vision
- Image IO: Handle reading, writing, even multiple images at once.
- Edge Detection: Crunch pixels and create sharp cutouts.
- Face Detection: From simple bounding boxes to naming who’s who and slapping on makeup filters, OpenCV can do it all.
SimpleCV: For Absolute Beginners
No PhD required. SimpleCV lets newbies run high-powered image processing—built right on OpenCV—without a single headache.
Real Example: The “Pedestrian Walk Sign” app tells you to "go" unless it detects a bright light (then it flips to "stop"). Yes, there’s a catch: SimpleCV is stuck on Python 2.7, but it’s still the fastest way for a rookie to play with computer vision.
Python GUI Libraries: Tkinter, WX Python & PyQt Face Off
Tkinter: Fast GUIs for Everyday Apps
Got a side project? Tkinter is the built-in Python GUI king for quick, simple app layouts. Drag-and-drop frames, labels, buttons—and customize everything from size to borders in minutes.
WX Python: The Cross-Platform Favorite
Pick WX Python when you need your GUI to run on Mac, Windows, AND Linux—without rewriting a line. Plus, you get gorgeous layouts, not “Windows 98” vibes.
Pro Example: “Outweicher” stores notes in a visual tree—built with WX Python.
PyQt: The Ultimate in Power & Flexibility
Want a web browser, help system, support for Unicode, regex, SQL databases, AND XML, all baked into your app? PyQt is your ultra-flexible weapon.
Real-World Builds: Calculators, weather dashboards, even crypto trackers.
Coding Productivity: Kite, The AI Autocomplete That’s Freakishly Good
What if your code editor finished your thoughts? Kite is a game-changing plugin—powered by AI—that saves you from typing out every tedious line. Atom, VS Code, Spider, PyCharm, Sublime, or Vim? Kite fits right in.
- Intelligent Snippets: Auto-completes even method arguments, not just function names.
- Kite Copilot: Live Python docs pop up next to your code—no more endless Googling.
- Totally Free Forever: Download, use, and accelerate your workflow instantly.
Game Development: Pygame, Piglet, and PyEngine3D
Why Every Programmer Should Try Game Dev—At Least Once
Building Pong or Tetris? Flex your creativity with these libraries:
- Pygame: The fastest way to build 2D games—with sound, animation, MIDI, collision detection, pixel manipulation, and wild browser support. Even runs on Android!
- Piglet: No external installs. Build 3D games and GUIs. Loads sound, video, images, and multiple windows out of the box.
- PyEngine3D: Open-source, pro-level graphics rendering—bring your 3D game ideas to life.
Quick Win: Browse thousands of community-built Pygame demos for endless inspiration.
Web Powerhouses: HTTP, Scraping, and Serverless Python
Requests: Sending HTTP Requests Like a Pro
If you’re not using Requests for APIs, you’re suffering unnecessarily. Send GET, POST, handle headers, form data, and multi-part files—it's $0, zero drama.
Scraping Libraries: Scrapy & BeautifulSoup
Scrapy: The go-to for building web crawlers that scrape, mine data, and auto test web flows. Think you don’t need scrapers? CareerBuilder, Parsely, Lish—they all rely on it.
BeautifulSoup: Best for quick-and-dirty HTML and XML parsing. Even if your HTML is an unholy mess, BeautifulSoup extracts what you need without a sweat.
Zappa: Effortless Serverless Apps on AWS Lambda
Serverless is the future. Zappa lets you host Python web apps that scale infinitely, cost pennies, and never go down. Build, deploy, forget—no devops nightmare.
Django vs. Flask: The Web Framework Deathmatch
- Django: Fastest way to ship robust, database-heavy websites. Handles auth, admin, sitemaps, feeds, and scales up easily. Used by Instagram, YouTube, Robinhood.
- Flask: Hotter than Django for smaller projects. More flexible, less boilerplate. Used by Airbnb, Netflix, Uber. Comes with built-in URL routing, debugging, and unit testing.
- Rule of Thumb: Heavy lifting? Go Django. Quick prototypes? Go Flask.
Mathematics and Data Science: NumPy, SciPy, SymPy and Beyond
NumPy: The Foundation of Python Data
Crunch numbers, wrangle matrices, run logical operations, Fourier transforms, linear algebra—all with blazing speed. NumPy is the core of every data science pipeline.
SciPy: Math, Machine Learning and More—Done Right
When you need integration, optimization, interpolation, or solid statistics—SciPy delivers. The community support is legendary (“There are no stupid questions” is the rule).
SymPy: Symbolic Math Without Tears
Calculus, Taylor series, matrix inversion, cryptography—stop using calculators or random web tools. SymPy makes tough math child’s play. Power apps like Spyder and Kempy leverage SymPy; you should too.
Pandas: The Data Science Juggernaut
Want to organize, manipulate, and visualize any data? Pandas is non-negotiable. Clean, intuitive, and shockingly powerful—makes data analysis a breeze even for total beginners.
Pro tip: Explore Orange for simple visuals, and SQLAlchemy if you’re moving data in and out of SQL databases.
Data Visualization and Machine Learning: Matplotlib, Plotly, Scikit-Learn, LightGBM, and More
Matplotlib: Plotting Like a Scientist
- Chart Types Galore: Histograms, scatter, pie, stream charts, you name it.
- GitHub Issue Tracker: Stay up to date with patches and bugs direct from the core team.
Plotly: Interactive, Web-Ready Visualization
Fluid, complex, and multi-axis plots with zoom, filter, and 3D. If Matplotlib feels too basic, graduate to Plotly.
Scikit-Learn: The Machine Learning Workhorse
- Classification, regression, clustering, dimensionality reduction, model selection, preprocessing—and that’s just the greatest hits.
- Slick syntax, tons of tutorials, rock-solid documentation.
Imbalanced-Learn: Fix Your Data Bias Fast
Are you getting 80% accuracy… because 80% of your data is the same class? Imbalanced-Learn is your fix, with clever resampling that pairs perfectly with Scikit-Learn.
Breakthroughs: LightGBM, LIME & More
- LightGBM: Gradient boosting that’s fast, memory-light, and brutally accurate. Leaf-wise tree growth is the secret sauce.
- LIME: Want to actually understand your classifier—globally and for individual predictions? LIME explains models in simple English, not just code.
“Most people never debug their models—then wonder why they fail.” Don’t be like most people.
Deep Learning Libraries: Keras, TensorFlow, PyTorch
Keras: Lightning-Fast Deep Learning Prototyping
- Build CNNs, RNNs: Both convolutional and recurrent networks.
- Run Anywhere: Use CPU, GPU, or even ship models to Android/iOS devices.
- Real Use: Image-to-HTML converters built in a weekend.
TensorFlow: End-to-End AI. No More Excuses
Intuitive APIs, model exports, debugging tools, runs on the cloud or directly in your browser—all under one massive (but approachable) roof.
- Build, train, and deploy models anywhere you want—no extra setup.
- Image Segmentation & Bounding Box Apps: Built from scratch using nothing but TensorFlow (and some grit).
PyTorch: R&D’s Favorite Framework
- GPU-Accelerated: Wildly fast tensor math.
- Used by Top Teams: Facebook AI Research, Uber Pyro.
- Huge Community: Get answers fast, share projects, and level up daily.
“Stop trying to be perfect. Start trying to be remarkable.”
Unique & Must-Know Python Libraries That Fly Under the Radar
- Twisted: The backbone of async network programming in Python. Used everywhere from Twisted Web to Twitch’s chat backend.
- IPython: The ultimate interactive shell—the engine behind Jupyter notebooks. Powerful for parallel computing workflows.
- Pillow: Take full control of any image file type (PDF, PNG, JPEG, GIF) with this “modern PIL.” Edit, blur, watermark, and never battle outdated docs again.
Productivity and Desktop Powerhouses: Poetry, Pywin32, Kivy, Pendulum, Loguru
- Poetry: Forget dependency nightmares. Manage, publish, and resolve package chaos in seconds.
- Pywin32: Automate Excel, Outlook, and any Windows app. Open, move, or copy data like a wizard.
- Kivy: Cross-platform UI and multi-touch for rapid mobile and desktop app development. Licensed MIT—build your next startup risk-free.
- Pendulum: Date/time math so smooth you’ll never write custom time conversion code again.
- Loguru: One line logging, color, and debug features so smart you’ll wonder how you ever used
print()
.
People Also Ask: Python Library Essentials
Which Python library should every beginner learn first?
Start with Numpy and Pandas for data, Requests for basic web work, Tkinter for GUIs, and Pygame for fun projects. These libraries give you the superpowers needed in most coding scenarios.
What is the most popular Python library in 2025?
Pandas and Scikit-Learn continue to dominate data science, while Requests is the “it” library for anything web-based. If you want fast growth, master at least two of these.
How do I choose the right Python library for my project?
Work backwards: What’s your end goal? Are you crunching data, building apps, or scraping the web? Read real-world case studies, test speed and compatibility, and always check for community and documentation quality.
Can I use Python libraries cross-platform?
Most major libraries—like Flask, Pygame, Kivy—work across Windows, Mac, and Linux. Always check dependencies, but Python’s ecosystem is more portable than almost any rival.
What are some hidden-gem Python libraries most pros use?
FlashText for fast keyword extraction, Loguru for logging, Pendulum for time handling, and IPython for interactive workflows rarely get the attention they deserve—but can instantly supercharge your projects.
Where to Go Next (Don’t Stop Here…)
Bottom Line: The Power’s in Your Hands Now
Most people will skim this and never take action. That’s why they stay stuck. But if you’re still reading, you’re already miles ahead—even if you just install one new library this week. The difference between winners and losers? Winners do what losers won’t.
Don’t just bookmark this—share it, quote it, and put these libraries into work. The faster you start, the faster you win. Need more arsenal? Check out the resources above, and get coding today.